using Pumas
using PumasUtilities
using Random
using CairoMakie
using AlgebraOfGraphics
using DataFramesMeta
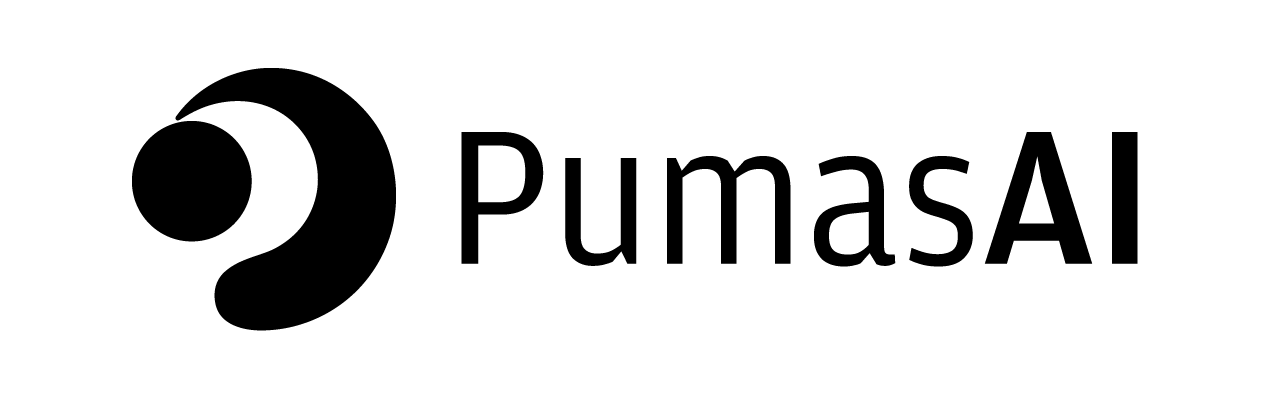
PK05 - One-compartment intravenous plasma/urine I
1 Learning Outcome
In this model, both plasma and urine data are collected, which will help to estimate parameters like Clearance, Volume of Distribution and fraction of dose excreted unchanged in urine.
2 Objectives
In this tutorial, you will learn how to:
- Build a one compartment model of a medication that undergoes excretion via urine
- Simulate the model for a single subject and a single dosing regimen, and
- Fit the model to data containing urine sample information
3 Background
Before constructing a model, it is important to establish the process the model will follow and a scenario for the simulation.
Below is the scenario for this tutorial:
- Structural Model - One Compartment Model with urinary excretion
- Route of Administration - Intravenous Bolus
- Dosage Regimen - 250 mg IV Bolus
- Subject - 1
This diagram describes how such an administered dose will be handled, which facilitates building the model.
4 Libraries
Call the required libraries to get started.
5 Model
In this one compartment model, we administer an IV dose in the central compartment.
@dynamics
In the @dynamics
block, a Urine Compartment is included. This measures the rate of change in amount over time and calculates the cumulative amount of drug in urine.
= @model begin
pk_05 @metadata begin
= "One Compartment Model with Urine Compartment"
desc = u"hr"
timeu end
@param begin
"""
Volume of Distribution (L)
"""
∈ RealDomain(lower = 0)
tvvc """
Renal Clearance(L/hr)
"""
∈ RealDomain(lower = 0)
tvClr """
Non Renal Clearance(L/hr)
"""
∈ RealDomain(lower = 0)
tvClnr ∈ PDiagDomain(3)
Ω """
Proportional RUV - Plasma
"""
∈ RealDomain(lower = 0)
σ_prop """
Additive RUV - Urine
"""
∈ RealDomain(lower = 0)
σ_add end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvClr * exp(η[1])
Clr = tvClnr * exp(η[2])
Clnr = tvvc * exp(η[3])
Vc end
@dynamics begin
' = -(Clnr / Vc) * Central - (Clr / Vc) * Central
Central' = (Clr / Vc) * Central
Urineend
@derived begin
"""
PK05 Plasma Concentration (mg/L)"
"""
= @. Central / Vc
cp_plasma ~ @. Normal(cp_plasma, abs(cp_plasma) * σ_prop)
dv_plasma
"""
PK05 Urine Amount (mg)
"""
= @. Urine
cp_urine ~ @. Normal(cp_urine, abs(σ_add))
dv_urine end
end
PumasModel
Parameters: tvvc, tvClr, tvClnr, Ω, σ_prop, σ_add
Random effects: η
Covariates:
Dynamical system variables: Central, Urine
Dynamical system type: Matrix exponential
Derived: cp_plasma, dv_plasma, cp_urine, dv_urine
Observed: cp_plasma, dv_plasma, cp_urine, dv_urine
6 Parameters
The parameters to define and estimate using the model are as mentioned below:
Clnr
- Non renal Clearance (L/hr)Clr
- Renal Clearance (L/hr)Vc
- Volume of the Central Compartment(L)Ω
- Between subject variabilityσ
- Residual Error
These are the initial estimates we will be using in this model exercise. Note that tv
represents the typical value for parameters.
= (;
param = 10.7965,
tvvc = 0.430905,
tvClr = 0.779591,
tvClnr = Diagonal([0.01, 0.01, 0.01]),
Ω = 0.1,
σ_prop = 1,
σ_add )
7 Dosage Regimen
To start the simulation process, the dosing regimen from the background section must be developed first. From our background, we established the scenario as a single dose of 250 mg given as an Intravenous bolus to a single subject.
The DosageRegimen
is specified as:
= DosageRegimen(250; time = 0, cmt = 1) ev1
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 250.0 | 1 | 0.0 | 0 | 0.0 | 0.0 | 0 | NullRoute |
This is how to create the single subject undergoing the dosing regimen above.
= Subject(;
sub = 1,
id = ev1,
events = (cp_plasma = nothing, cp_urine = nothing),
observations )
Subject
ID: 1
Events: 1
Observations: cp_plasma: (n=0), cp_urine: (n=0)
8 Simulation
Let’s simulate the plasma concentration and the unchanged amount excreted in urine.
The Random.seed!
function is included here for purposes of reproducibility of the simulation in this tutorial. Specification of a seed value would not be required in a Pumas workflow that is estimating model parameters.
Random.seed!(123)
= simobs(pk_05, sub, param, obstimes = 0:0.1:26) sim_sub1
SimulatedObservations
Simulated variables: cp_plasma, dv_plasma, cp_urine, dv_urine
Time: 0.0:0.1:26.0
9 Visualization
These figures display the drug concentration levels in both plasma and urine samples.
= renamer(
variable_renamer "cp_plasma" => "Plasma \n concentration (mg/L)",
"cp_urine" => "Urine \n amount (mg)",
)
= @chain DataFrame(sim_sub1) begin
plot_df @select :id :time :cp_plasma :cp_urine
dropmissing([:cp_plasma, :cp_urine])
stack(Not(:id, :time))
end
=
plt_rows data(plot_df) *
mapping(:time => "Time (hours)", :value => "", row = :variable => variable_renamer) *
visual(Lines; linewidth = 4)
draw(plt_rows; figure = (; fontsize = 22), facet = (; linkyaxes = :none))
By looking at the figure, we can observe that the drug level is decreasing in plasma and increasing in the urine, which resembles normal physiologic function.
When we put the visualization together, we observe the following figure:
=
plt_join data(plot_df) *
mapping(
:time => "Time (hours)",
:value => "Concentration / Amount",
= :variable => variable_renamer => "",
color *
) visual(Lines; linewidth = 4)
draw(plt_join; figure = (; fontsize = 22), legend = (; position = :bottom))
10 Population Simulation
This block updates the parameters of the model to increase intersubject variability in parameters and defines timepoints for prediction of concentrations. The results are written to a CSV file.
= (
par = 10.7965,
tvvc = 0.430905,
tvClr = 0.779591,
tvClnr = Diagonal([0.04, 0.09, 0.0225]),
Ω = 0.0256,
σ_prop = 3.126,
σ_add
)
= DosageRegimen(250; time = 0, cmt = 1)
ev1 = map(i -> Subject(id = i, events = ev1), 1:55)
pop
Random.seed!(1234)
= simobs(pk_05, pop, par, obstimes = [0.5, 1, 1.5, 2, 4, 6, 8, 12, 18, 24])
pop_sim sim_plot(pop_sim)
= DataFrame(pop_sim)
df_sim
#CSV.write("pk_05.csv", df_sim)
With the CSV.write
function, you can input the name of the dataframe (df_sim
) and the file name of your choice (pk_05.csv
) to save the file to your local directory or repository.
11 Conclusion
Constructing a one compartment model with urine elimination involves:
- understanding the process of how the drug is passed through the system,
- translating processes into ODEs using Pumas,
- explaining the drug elimination process in Pumas, and
- simulating the model in a single patient for evaluation.