using Pumas
using PumasUtilities
using NCA
using NCAUtilities
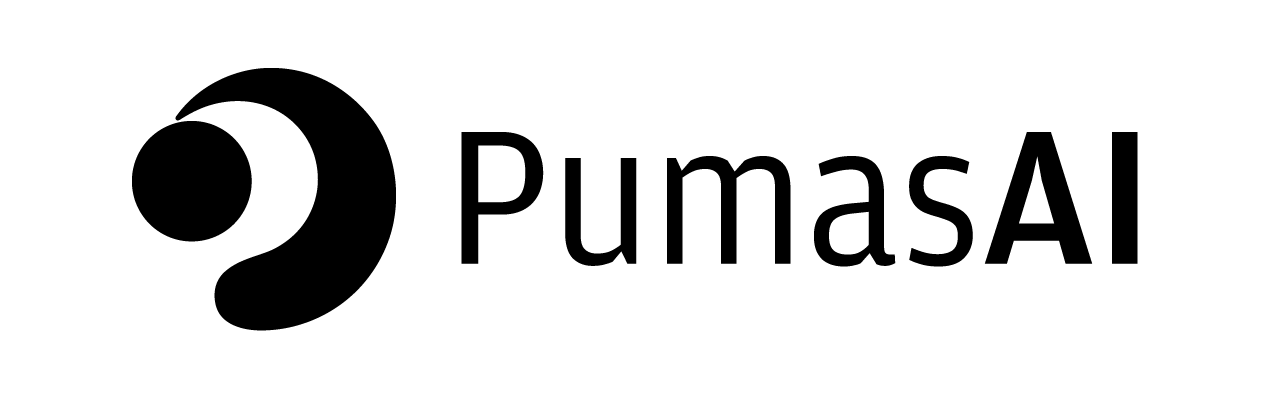
A Comprehensive Introduction to Pumas
This tutorial provides a comprehensive introduction to a modeling and simulation workflow in Pumas. The idea is not to get into the details of Pumas specifics, but instead provide a narrative on the lines of a regular workflow in our day-to-day work, with brevity where required to allow a broad overview. Wherever possible, cross-references will be provided to documentation and detailed examples that provide deeper insight into a particular topic.
As part of this workflow, you will be introduced to various aspects such as:
- Data wrangling in Julia
- Exploratory analysis in Julia
- Continuous data non-linear mixed effects modeling in Pumas
- Model comparison routines, post-processing, validation etc.
1 The Study and Design
CTMNopain is a novel anti-inflammatory agent under preliminary investigation. A dose-ranging trial was conducted comparing placebo with 3 doses of CTMNopain (5mg, 20mg and 80 mg QD). The maximum tolerated dose is 160 mg per day. Plasma concentrations (mg/L) of the drug were measured at 0
, 0.5
, 1
, 1.5
, 2
, 2.5
, 3
-8
hours.
Pain score (0
=no pain, 1
=mild, 2
=moderate, 3
=severe) were obtained at time points when plasma concentration was collected. A pain score of 2
or more is considered as no pain relief.
The subjects can request for remedication if pain relief is not achieved after 2 hours post dose. Some subjects had remedication before 2 hours if they were not able to bear the pain. The time to remedication and the remedication status is available for subjects.
The pharmacokinetic dataset can be accessed using PharmaDatasets.jl
.
2 Setup
2.1 Load libraries
These libraries provide the workhorse functionality in the Pumas ecosystem:
In addition, libraries below are good add-on’s that provide ancillary functionality:
using GLM: lm, @formula
using Random
using CSV
using DataFramesMeta
using CairoMakie
using PharmaDatasets
2.2 Data Wrangling
We start by reading in the dataset and making some quick summaries.
If you want to learn more about data wrangling, don’t forget to check our Data Wrangling in Julia tutorials!
= dataset("pk_painrelief")
pkpain_df first(pkpain_df, 5)
Row | Subject | Time | Conc | PainRelief | PainScore | RemedStatus | Dose |
---|---|---|---|---|---|---|---|
Int64 | Float64 | Float64 | Int64 | Int64 | Int64 | String7 | |
1 | 1 | 0.0 | 0.0 | 0 | 3 | 1 | 20 mg |
2 | 1 | 0.5 | 1.15578 | 1 | 1 | 0 | 20 mg |
3 | 1 | 1.0 | 1.37211 | 1 | 0 | 0 | 20 mg |
4 | 1 | 1.5 | 1.30058 | 1 | 0 | 0 | 20 mg |
5 | 1 | 2.0 | 1.19195 | 1 | 1 | 0 | 20 mg |
Let’s filter out the placebo data as we don’t need that for the PK analysis.
= @rsubset pkpain_df :Dose != "Placebo";
pkpain_noplb_df first(pkpain_noplb_df, 5)
Row | Subject | Time | Conc | PainRelief | PainScore | RemedStatus | Dose |
---|---|---|---|---|---|---|---|
Int64 | Float64 | Float64 | Int64 | Int64 | Int64 | String7 | |
1 | 1 | 0.0 | 0.0 | 0 | 3 | 1 | 20 mg |
2 | 1 | 0.5 | 1.15578 | 1 | 1 | 0 | 20 mg |
3 | 1 | 1.0 | 1.37211 | 1 | 0 | 0 | 20 mg |
4 | 1 | 1.5 | 1.30058 | 1 | 0 | 0 | 20 mg |
5 | 1 | 2.0 | 1.19195 | 1 | 1 | 0 | 20 mg |
3 Analysis
3.1 Non-compartmental analysis
Let’s begin by performing a quick NCA of the concentration time profiles and view the exposure changes across doses. The input data specification for NCA analysis requires the presence of a :route
column and an :amt
column that specifies the dose. So, let’s add that in:
@rtransform! pkpain_noplb_df begin
:route = "ev"
:Dose = parse(Int, chop(:Dose; tail = 3))
end
We also need to create an :amt
column:
@rtransform! pkpain_noplb_df :amt = :Time == 0 ? :Dose : missing
Now, we map the data variables to the read_nca
function that prepares the data for NCA analysis.
= read_nca(
pkpain_nca
pkpain_noplb_df;= :Subject,
id = :Time,
time = :amt,
amt = :Conc,
observations = [:Dose],
group = :route,
route )
NCAPopulation (120 subjects):
Group: [["Dose" => 5], ["Dose" => 20], ["Dose" => 80]]
Number of missing observations: 0
Number of blq observations: 0
Now that we mapped the data in, let’s visualize the concentration vs time plots for a few individuals. When paginate
is set to true
, a vector of plots are returned and below we display the first element with 9 individuals.
= observations_vs_time(
f
pkpain_nca;= true,
paginate = (; xlabel = "Time (hr)", ylabel = "CTMNoPain Concentration (ng/mL)"),
axis = (; combinelabels = true),
facet
)1] f[
or you can view the summary curves by dose group as passed in to the group
argument in read_nca
summary_observations_vs_time(
pkpain_nca,= (; fontsize = 22, size = (800, 1000)),
figure = "black",
color = 3,
linewidth = (; xlabel = "Time (hr)", ylabel = "CTMX Concentration (μg/mL)"),
axis = (; combinelabels = true, linkaxes = true),
facet )
A full NCA Report is now obtained for completeness purposes using the run_nca
function, but later we will only extract a couple of key metrics of interest.
= run_nca(pkpain_nca; sigdigits = 3) pk_nca
We can look at the NCA fits for some subjects. Here f
is a vector or figures. We’ll showcase the first image by indexing f
:
= subject_fits(
f
pk_nca,= true,
paginate = (; xlabel = "Time (hr)", ylabel = "CTMX Concentration (μg/mL)"),
axis = (; combinelabels = true, linkaxes = true),
facet
)1] f[
As CTMNopain’s effect maybe mainly related to maximum concentration (cmax
) or area under the curve (auc
), we present some summary statistics using the summarize
function from NCA
.
= [:Dose] strata
1-element Vector{Symbol}:
:Dose
= [:cmax, :aucinf_obs] params
2-element Vector{Symbol}:
:cmax
:aucinf_obs
= summarize(pk_nca; stratify_by = strata, parameters = params) output
Row | Dose | parameters | numsamples | minimum | maximum | mean | std | geomean | geostd | geomeanCV |
---|---|---|---|---|---|---|---|---|---|---|
Int64 | String | Int64 | Float64 | Float64 | Float64 | Float64 | Float64 | Float64 | Float64 | |
1 | 5 | cmax | 40 | 0.19 | 0.539 | 0.356075 | 0.0884129 | 0.345104 | 1.2932 | 26.1425 |
2 | 5 | aucinf_obs | 40 | 0.914 | 3.4 | 1.5979 | 0.490197 | 1.53373 | 1.32974 | 29.0868 |
3 | 20 | cmax | 40 | 0.933 | 2.7 | 1.4737 | 0.361871 | 1.43408 | 1.2633 | 23.6954 |
4 | 20 | aucinf_obs | 40 | 2.77 | 14.1 | 6.377 | 2.22239 | 6.02031 | 1.41363 | 35.6797 |
5 | 80 | cmax | 40 | 3.3 | 8.47 | 5.787 | 1.31957 | 5.64164 | 1.25757 | 23.2228 |
6 | 80 | aucinf_obs | 40 | 13.7 | 49.1 | 29.5 | 8.68984 | 28.2954 | 1.34152 | 30.0258 |
The statistics printed above are the default, but you can pass in your own statistics using the stats = []
argument to the summarize
function.
We can look at a few parameter distribution plots.
parameters_vs_group(
pk_nca,= :cmax,
parameter = (; xlabel = "Dose (mg)", ylabel = "Cₘₐₓ (ng/mL)"),
axis = (; fontsize = 18),
figure )
Dose normalized PK parameters, cmax
and aucinf
were essentially dose proportional between for 5 mg, 20 mg and 80 mg doses. You can perform a simple regression to check the impact of dose on cmax
:
= NCA.DoseLinearityPowerModel(pk_nca, :cmax; level = 0.9) dp
Dose Linearity Power Model
Variable: cmax
Model: log(cmax) ~ log(α) + β × log(dose)
────────────────────────────────────
Estimate low CI 90% high CI 90%
────────────────────────────────────
β 1.00775 0.97571 1.0398
────────────────────────────────────
Here’s a visualization for the dose linearity using a power model for cmax
:
power_model(dp)
We can also visualize a dose proportionality results with respect to a specific endpoint in a NCA Report; for example cmax
and aucinf_obs
:
dose_vs_dose_normalized(pk_nca, :cmax)
dose_vs_dose_normalized(pk_nca, :aucinf_obs)
Based on visual inspection of the concentration time profiles as seen earlier, CTMNopain exhibited monophasic decline, and perhaps a one compartment model best fits the PK data.
3.2 Pharmacokinetic modeling
As seen from the plots above, the concentrations decline monoexponentially. We will evaluate both one and two compartment structural models to assess best fit. Further, different residual error models will also be tested.
We will use the results from NCA to provide us good initial estimates.
3.2.1 Data preparation for modeling
PumasNDF requires the presence of :evid
and :cmt
columns in the dataset.
@rtransform! pkpain_noplb_df begin
:evid = :Time == 0 ? 1 : 0
:cmt = :Time == 0 ? 1 : 2
:cmt2 = 1 # for zero order absorption
end
Further, observations at time of dosing, i.e., when evid = 1
have to be missing
@rtransform! pkpain_noplb_df :Conc = :evid == 1 ? missing : :Conc
The dataframe will now be converted to a Population
using read_pumas
. Note that both observations
and covariates
are required to be an array even if it is one element.
= read_pumas(
pkpain_noplb
pkpain_noplb_df;= :Subject,
id = :Time,
time = :amt,
amt = [:Conc],
observations = [:Dose],
covariates = :evid,
evid = :cmt,
cmt )
Population
Subjects: 120
Covariates: Dose
Observations: Conc
Now that the data is transformed to a Population
of subjects, we can explore different models.
3.2.2 One-compartment model
If you are not familiar yet with the @model
blocks and syntax, please check our documentation.
= @model begin
pk_1cmp
@metadata begin
= "One Compartment Model"
desc = u"hr"
timeu end
@param begin
"""
Clearance (L/hr)
"""
∈ RealDomain(; lower = 0, init = 3.2)
tvcl """
Volume (L)
"""
∈ RealDomain(; lower = 0, init = 16.4)
tvv """
Absorption rate constant (h-1)
"""
∈ RealDomain(; lower = 0, init = 3.8)
tvka """
- ΩCL
- ΩVc
- ΩKa
"""
∈ PDiagDomain(init = [0.04, 0.04, 0.04])
Ω """
Proportional RUV
"""
∈ RealDomain(; lower = 0.0001, init = 0.2)
σ_p end
@random begin
~ MvNormal(Ω)
η end
@covariates begin
"""
Dose (mg)
"""
Doseend
@pre begin
= tvcl * exp(η[1])
CL = tvv * exp(η[2])
Vc = tvka * exp(η[3])
Ka end
@dynamics Depots1Central1
@derived begin
:= @. Central / Vc
cp """
CTMx Concentration (ng/mL)
"""
~ @. Normal(cp, abs(cp) * σ_p)
Conc end
end
┌ Warning: Covariate Dose is not used in the model.
└ @ Pumas ~/run/_work/PumasTutorials.jl/PumasTutorials.jl/custom_julia_depot/packages/Pumas/GJfPM/src/dsl/model_macro.jl:2958
PumasModel
Parameters: tvcl, tvv, tvka, Ω, σ_p
Random effects: η
Covariates: Dose
Dynamical system variables: Depot, Central
Dynamical system type: Closed form
Derived: Conc
Observed: Conc
Note that the local assignment :=
can be used to define intermediate statements that will not be carried outside of the block. This means that all the resulting data workflows from this model will not contain the intermediate variables defined with :=
. We use this when we want to suppress the variable from any further output.
The idea behind :=
is for performance reasons. If you are not carrying the variable defined with :=
outside of the block, then it is not necessary to store it in the resulting data structures. Not only will your model run faster, but the resulting data structures will also be smaller.
Before going to fit the model, let’s evaluate some helpful steps via simulation to check appropriateness of data and model
# zero out the random effects
= zero_randeffs(pk_1cmp, init_params(pk_1cmp), pkpain_noplb) etas
Above, we are generating a vector of η
’s of the same length as the number of subjects to zero out the random effects. We do this as we are evaluating the trajectories of the concentrations at the initial set of parameters at a population level. Other helper functions here are sample_randeffs
and init_randeffs
. Please refer to the documentation.
= simobs(pk_1cmp, pkpain_noplb, init_params(pk_1cmp), etas) simpk_iparams
Simulated population (Vector{<:Subject})
Simulated subjects: 120
Simulated variables: Conc
sim_plot(
pk_1cmp,
simpk_iparams;= [:Conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Observed/Predicted \n CTMx Concentration (ng/mL)",
ylabel
), )
Our NCA based initial guess on the parameters seem to work well.
Lets change the initial estimate of a couple of the parameters to evaluate the sensitivity.
= (; init_params(pk_1cmp)..., tvka = 2, tvv = 10) pkparam
(tvcl = 3.2,
tvv = 10,
tvka = 2,
Ω = [0.04 0.0 0.0; 0.0 0.04 0.0; 0.0 0.0 0.04],
σ_p = 0.2,)
= simobs(pk_1cmp, pkpain_noplb, pkparam, etas) simpk_changedpars
Simulated population (Vector{<:Subject})
Simulated subjects: 120
Simulated variables: Conc
sim_plot(
pk_1cmp,
simpk_changedpars;= [:Conc],
observations = (; fontsize = 18),
figure = (
axis = "Time (hr)",
xlabel = "Observed/Predicted \n CTMx Concentration (ng/mL)",
ylabel
), )
Changing the tvka
and decreasing the tvv
seemed to make an impact and observations go through the simulated lines.
To get a quick ballpark estimate of your PK parameters, we can do a NaivePooled
analysis.
3.2.2.1 NaivePooled
= fit(pk_1cmp, pkpain_noplb, init_params(pk_1cmp), NaivePooled(); omegas = (:Ω,)) pkfit_np
[ Info: Checking the initial parameter values.
[ Info: The initial negative log likelihood and its gradient are finite. Check passed.
Iter Function value Gradient norm
0 7.744356e+02 3.715711e+03
* time: 0.022976160049438477
1 2.343899e+02 1.747348e+03
* time: 1.0286791324615479
2 9.696232e+01 1.198088e+03
* time: 1.0320501327514648
3 -7.818699e+01 5.538151e+02
* time: 1.0342581272125244
4 -1.234803e+02 2.462514e+02
* time: 1.0363490581512451
5 -1.372888e+02 2.067458e+02
* time: 1.0385570526123047
6 -1.410579e+02 1.162950e+02
* time: 1.0408351421356201
7 -1.434754e+02 5.632816e+01
* time: 1.0433530807495117
8 -1.453401e+02 7.859270e+01
* time: 1.0461151599884033
9 -1.498185e+02 1.455606e+02
* time: 1.0487091541290283
10 -1.534371e+02 1.303682e+02
* time: 1.0511481761932373
11 -1.563557e+02 5.975474e+01
* time: 1.0532500743865967
12 -1.575052e+02 9.308611e+00
* time: 1.0552170276641846
13 -1.579357e+02 1.234484e+01
* time: 1.0574631690979004
14 -1.581874e+02 7.478196e+00
* time: 1.0595932006835938
15 -1.582981e+02 2.027162e+00
* time: 1.0615789890289307
16 -1.583375e+02 5.578262e+00
* time: 1.0635449886322021
17 -1.583556e+02 4.727050e+00
* time: 1.0660309791564941
18 -1.583644e+02 2.340173e+00
* time: 1.0684270858764648
19 -1.583680e+02 7.738100e-01
* time: 1.0709881782531738
20 -1.583696e+02 3.300689e-01
* time: 1.0735750198364258
21 -1.583704e+02 3.641985e-01
* time: 1.0762059688568115
22 -1.583707e+02 4.365901e-01
* time: 1.0787920951843262
23 -1.583709e+02 3.887800e-01
* time: 1.0814120769500732
24 -1.583710e+02 2.766977e-01
* time: 1.084015130996704
25 -1.583710e+02 1.758029e-01
* time: 1.0865700244903564
26 -1.583710e+02 1.133947e-01
* time: 1.0890581607818604
27 -1.583710e+02 7.922544e-02
* time: 1.0915031433105469
28 -1.583710e+02 5.954998e-02
* time: 1.0934860706329346
29 -1.583710e+02 4.157079e-02
* time: 1.0954620838165283
30 -1.583710e+02 4.295447e-02
* time: 1.0977931022644043
31 -1.583710e+02 5.170753e-02
* time: 1.1003830432891846
32 -1.583710e+02 2.644383e-02
* time: 1.103865146636963
33 -1.583710e+02 4.548993e-03
* time: 1.1071829795837402
34 -1.583710e+02 2.501804e-02
* time: 1.1105570793151855
35 -1.583710e+02 3.763440e-02
* time: 1.113267183303833
36 -1.583710e+02 3.206026e-02
* time: 1.1159181594848633
37 -1.583710e+02 1.003698e-02
* time: 1.118575096130371
38 -1.583710e+02 2.209089e-02
* time: 1.1212060451507568
39 -1.583710e+02 4.954172e-03
* time: 1.123840093612671
40 -1.583710e+02 1.609373e-02
* time: 1.127295970916748
41 -1.583710e+02 1.579802e-02
* time: 1.129986047744751
42 -1.583710e+02 1.014113e-03
* time: 1.1325900554656982
43 -1.583710e+02 6.050644e-03
* time: 1.136044979095459
44 -1.583710e+02 1.354412e-02
* time: 1.1387221813201904
45 -1.583710e+02 4.473248e-03
* time: 1.1414051055908203
46 -1.583710e+02 4.644735e-03
* time: 1.1441171169281006
47 -1.583710e+02 9.829910e-03
* time: 1.146846055984497
48 -1.583710e+02 1.047561e-03
* time: 1.1495881080627441
49 -1.583710e+02 8.366895e-03
* time: 1.1522881984710693
50 -1.583710e+02 7.879055e-04
* time: 1.1550211906433105
FittedPumasModel
Successful minimization: true
Likelihood approximation: NaivePooled
Likelihood Optimizer: BFGS
Dynamical system type: Closed form
Log-likelihood value: 158.37103
Number of subjects: 120
Number of parameters: Fixed Optimized
1 6
Observation records: Active Missing
Conc: 1320 0
Total: 1320 0
------------------
Estimate
------------------
tvcl 3.0054
tvv 14.089
tvka 44.228
Ω₁,₁ 0.0
Ω₂,₂ 0.0
Ω₃,₃ 0.0
σ_p 0.32999
------------------
coefficients_table(pkfit_np)
Row | Parameter | Description | Estimate |
---|---|---|---|
String | Abstract… | Float64 | |
1 | tvcl | Clearance (L/hr)\n | 3.005 |
2 | tvv | Volume (L)\n | 14.089 |
3 | tvka | Absorption rate constant (h-1)\n | 44.228 |
4 | Ω₁,₁ | ΩCL | 0.0 |
5 | Ω₂,₂ | ΩVc | 0.0 |
6 | Ω₃,₃ | ΩKa | 0.0 |
7 | σ_p | Proportional RUV\n | 0.33 |
The final estimates from the NaivePooled
approach seem reasonably close to our initial guess from NCA, except for the tvka
parameter. We will stick with our initial guess.
One way to be cautious before going into a complete fit
ting routine is to evaluate the likelihood of the individual subjects given the initial parameter values and see if any subject(s) pops out as unreasonable. There are a few ways of doing this:
- check the
loglikelihood
subject wise - check if there any influential subjects
Below, we are basically checking if the initial estimates for any subject are way off that we are unable to compute the initial loglikelihood
.
= []
lls for subj in pkpain_noplb
push!(lls, loglikelihood(pk_1cmp, subj, pkparam, FOCE()))
end
# the plot below is using native CairoMakie `hist`
hist(lls; bins = 10, normalization = :none, color = (:black, 0.5))
The distribution of the loglikelihood’s suggest no extreme outliers.
A more convenient way is to use the findinfluential
function that provides a list of k
top influential subjects by showing the normalized (minus) loglikelihood for each subject. As you can see below, the minus loglikelihood in the range of 16 agrees with the histogram plotted above.
= findinfluential(pk_1cmp, pkpain_noplb, pkparam, FOCE()) influential_subjects
120-element Vector{@NamedTuple{id::String, nll::Float64}}:
(id = "148", nll = 16.65965885684477)
(id = "135", nll = 16.648985190076335)
(id = "156", nll = 15.959069556607496)
(id = "159", nll = 15.441218240496484)
(id = "149", nll = 14.71513464411951)
(id = "88", nll = 13.09709837464614)
(id = "16", nll = 12.98228052193144)
(id = "61", nll = 12.652182902303679)
(id = "71", nll = 12.500330088085505)
(id = "59", nll = 12.241510254805235)
⋮
(id = "57", nll = -22.79767423253431)
(id = "93", nll = -22.836900711478208)
(id = "12", nll = -23.007742339519247)
(id = "123", nll = -23.292751843079234)
(id = "41", nll = -23.425412534960515)
(id = "99", nll = -23.535214841901112)
(id = "29", nll = -24.025959868383083)
(id = "52", nll = -24.164757842493685)
(id = "24", nll = -25.57209232565845)
3.2.2.2 FOCE
Now that we have a good handle on our data, lets go ahead and fit
a population model with FOCE
:
= fit(pk_1cmp, pkpain_noplb, pkparam, FOCE(); constantcoef = (; tvka = 2)) pkfit_1cmp
[ Info: Checking the initial parameter values.
[ Info: The initial negative log likelihood and its gradient are finite. Check passed.
Iter Function value Gradient norm
0 -5.935351e+02 5.597318e+02
* time: 6.794929504394531e-5
1 -7.022088e+02 1.707063e+02
* time: 0.3063819408416748
2 -7.314067e+02 2.903269e+02
* time: 0.47862792015075684
3 -8.520591e+02 2.285888e+02
* time: 0.6429300308227539
4 -1.120191e+03 3.795410e+02
* time: 1.0446679592132568
5 -1.178784e+03 2.323978e+02
* time: 1.1877639293670654
6 -1.218320e+03 9.699907e+01
* time: 1.336503028869629
7 -1.223641e+03 5.862105e+01
* time: 1.4849469661712646
8 -1.227620e+03 1.831403e+01
* time: 1.6717040538787842
9 -1.228381e+03 2.132323e+01
* time: 1.7957069873809814
10 -1.230098e+03 2.921228e+01
* time: 1.9300000667572021
11 -1.230854e+03 2.029662e+01
* time: 2.0620429515838623
12 -1.231116e+03 5.229097e+00
* time: 2.186326026916504
13 -1.231179e+03 1.689232e+00
* time: 2.3499300479888916
14 -1.231187e+03 1.215379e+00
* time: 2.4502830505371094
15 -1.231188e+03 2.770380e-01
* time: 2.5491058826446533
16 -1.231188e+03 1.636653e-01
* time: 2.640552043914795
17 -1.231188e+03 2.701133e-01
* time: 2.7346630096435547
18 -1.231188e+03 3.163363e-01
* time: 2.829946994781494
19 -1.231188e+03 1.505149e-01
* time: 2.9668710231781006
20 -1.231188e+03 2.484999e-02
* time: 3.042357921600342
21 -1.231188e+03 8.446863e-04
* time: 3.114832878112793
FittedPumasModel
Successful minimization: true
Likelihood approximation: FOCE
Likelihood Optimizer: BFGS
Dynamical system type: Closed form
Log-likelihood value: 1231.188
Number of subjects: 120
Number of parameters: Fixed Optimized
1 6
Observation records: Active Missing
Conc: 1320 0
Total: 1320 0
-------------------
Estimate
-------------------
tvcl 3.1642
tvv 13.288
tvka 2.0
Ω₁,₁ 0.08494
Ω₂,₂ 0.048568
Ω₃,₃ 5.5811
σ_p 0.10093
-------------------
infer(pkfit_1cmp)
[ Info: Calculating: variance-covariance matrix.
[ Info: Done.
Asymptotic inference results using sandwich estimator
Successful minimization: true
Likelihood approximation: FOCE
Likelihood Optimizer: BFGS
Dynamical system type: Closed form
Log-likelihood value: 1231.188
Number of subjects: 120
Number of parameters: Fixed Optimized
1 6
Observation records: Active Missing
Conc: 1320 0
Total: 1320 0
-------------------------------------------------------------------
Estimate SE 95.0% C.I.
-------------------------------------------------------------------
tvcl 3.1642 0.08662 [ 2.9944 ; 3.334 ]
tvv 13.288 0.27481 [12.749 ; 13.827 ]
tvka 2.0 NaN [ NaN ; NaN ]
Ω₁,₁ 0.08494 0.011022 [ 0.063338; 0.10654 ]
Ω₂,₂ 0.048568 0.0063502 [ 0.036122; 0.061014]
Ω₃,₃ 5.5811 1.2188 [ 3.1922 ; 7.97 ]
σ_p 0.10093 0.0057196 [ 0.089718; 0.11214 ]
-------------------------------------------------------------------
Notice that tvka
is fixed to 2 as we don’t have a lot of information before tmax
. From the results above, we see that the parameter precision for this model is reasonable.
3.2.3 Two-compartment model
Just to be sure, let’s fit a 2-compartment model and evaluate:
= @model begin
pk_2cmp
@param begin
"""
Clearance (L/hr)
"""
∈ RealDomain(; lower = 0, init = 3.2)
tvcl """
Central Volume (L)
"""
∈ RealDomain(; lower = 0, init = 16.4)
tvv """
Peripheral Volume (L)
"""
∈ RealDomain(; lower = 0, init = 10)
tvvp """
Distributional Clearance (L/hr)
"""
∈ RealDomain(; lower = 0, init = 2)
tvq """
Absorption rate constant (h-1)
"""
∈ RealDomain(; lower = 0, init = 1.3)
tvka """
- ΩCL
- ΩVc
- ΩKa
- ΩVp
- ΩQ
"""
∈ PDiagDomain(init = [0.04, 0.04, 0.04, 0.04, 0.04])
Ω """
Proportional RUV
"""
∈ RealDomain(; lower = 0.0001, init = 0.2)
σ_p end
@random begin
~ MvNormal(Ω)
η end
@covariates begin
"""
Dose (mg)
"""
Doseend
@pre begin
= tvcl * exp(η[1])
CL = tvv * exp(η[2])
Vc = tvka * exp(η[3])
Ka = tvvp * exp(η[4])
Vp = tvq * exp(η[5])
Q end
@dynamics Depots1Central1Periph1
@derived begin
:= @. Central / Vc
cp """
CTMx Concentration (ng/mL)
"""
~ @. Normal(cp, cp * σ_p)
Conc end
end
┌ Warning: Covariate Dose is not used in the model.
└ @ Pumas ~/run/_work/PumasTutorials.jl/PumasTutorials.jl/custom_julia_depot/packages/Pumas/GJfPM/src/dsl/model_macro.jl:2958
PumasModel
Parameters: tvcl, tvv, tvvp, tvq, tvka, Ω, σ_p
Random effects: η
Covariates: Dose
Dynamical system variables: Depot, Central, Peripheral
Dynamical system type: Closed form
Derived: Conc
Observed: Conc
3.2.3.1 FOCE
=
pkfit_2cmp fit(pk_2cmp, pkpain_noplb, init_params(pk_2cmp), FOCE(); constantcoef = (; tvka = 2))
[ Info: Checking the initial parameter values.
[ Info: The initial negative log likelihood and its gradient are finite. Check passed.
Iter Function value Gradient norm
0 -6.302369e+02 1.021050e+03
* time: 6.914138793945312e-5
1 -9.197817e+02 9.927951e+02
* time: 0.32505202293395996
2 -1.372640e+03 2.054986e+02
* time: 0.6161291599273682
3 -1.446326e+03 1.543987e+02
* time: 0.8884520530700684
4 -1.545570e+03 1.855028e+02
* time: 1.1908230781555176
5 -1.581449e+03 1.713157e+02
* time: 1.6332471370697021
6 -1.639433e+03 1.257382e+02
* time: 1.9033780097961426
7 -1.695964e+03 7.450539e+01
* time: 2.2038440704345703
8 -1.722243e+03 5.961044e+01
* time: 2.483635187149048
9 -1.736883e+03 7.320921e+01
* time: 2.76632022857666
10 -1.753547e+03 7.501938e+01
* time: 3.0248570442199707
11 -1.764053e+03 6.185661e+01
* time: 3.3146021366119385
12 -1.778991e+03 4.831033e+01
* time: 3.621286153793335
13 -1.791492e+03 4.943278e+01
* time: 3.961350202560425
14 -1.799847e+03 2.871410e+01
* time: 4.313982009887695
15 -1.805374e+03 7.520791e+01
* time: 4.664659023284912
16 -1.816260e+03 2.990621e+01
* time: 5.001169204711914
17 -1.818252e+03 2.401915e+01
* time: 5.307007074356079
18 -1.822988e+03 2.587225e+01
* time: 5.627120018005371
19 -1.824653e+03 1.550517e+01
* time: 5.90357518196106
20 -1.826074e+03 1.788927e+01
* time: 6.217127084732056
21 -1.826821e+03 1.888389e+01
* time: 6.516906023025513
22 -1.827900e+03 1.432840e+01
* time: 6.811236143112183
23 -1.828511e+03 9.422041e+00
* time: 7.117821216583252
24 -1.828754e+03 5.363442e+00
* time: 7.436201095581055
25 -1.828862e+03 4.916159e+00
* time: 7.697673082351685
26 -1.829007e+03 4.695755e+00
* time: 7.98659610748291
27 -1.829358e+03 1.090249e+01
* time: 8.290166139602661
28 -1.829830e+03 1.451325e+01
* time: 8.60358214378357
29 -1.830201e+03 1.108715e+01
* time: 8.914958000183105
30 -1.830360e+03 2.891223e+00
* time: 9.224247217178345
31 -1.830390e+03 1.695557e+00
* time: 9.490147113800049
32 -1.830404e+03 1.601712e+00
* time: 9.794012069702148
33 -1.830432e+03 2.823385e+00
* time: 10.101286172866821
34 -1.830477e+03 4.060617e+00
* time: 10.4063081741333
35 -1.830528e+03 5.133499e+00
* time: 10.716417074203491
36 -1.830593e+03 2.830970e+00
* time: 11.036266088485718
37 -1.830616e+03 3.342835e+00
* time: 11.349440097808838
38 -1.830622e+03 3.708884e+00
* time: 11.624987125396729
39 -1.830625e+03 2.062934e+00
* time: 11.921450138092041
40 -1.830627e+03 1.278569e+00
* time: 12.19426703453064
41 -1.830628e+03 1.832895e+00
* time: 12.495031118392944
42 -1.830628e+03 3.768840e-01
* time: 12.804509162902832
43 -1.830629e+03 3.152895e-01
* time: 13.045242071151733
44 -1.830630e+03 4.871060e-01
* time: 13.371952056884766
45 -1.830630e+03 3.110627e-01
* time: 13.683919191360474
46 -1.830630e+03 2.687758e-02
* time: 13.978915214538574
47 -1.830630e+03 4.694018e-03
* time: 14.18165922164917
48 -1.830630e+03 8.272969e-03
* time: 14.39710021018982
49 -1.830630e+03 8.249151e-03
* time: 14.665932178497314
50 -1.830630e+03 8.245562e-03
* time: 14.997864007949829
51 -1.830630e+03 8.240030e-03
* time: 15.338874101638794
52 -1.830630e+03 8.240030e-03
* time: 15.708791017532349
53 -1.830630e+03 8.240030e-03
* time: 16.07951521873474
FittedPumasModel
Successful minimization: true
Likelihood approximation: FOCE
Likelihood Optimizer: BFGS
Dynamical system type: Closed form
Log-likelihood value: 1830.6304
Number of subjects: 120
Number of parameters: Fixed Optimized
1 10
Observation records: Active Missing
Conc: 1320 0
Total: 1320 0
-------------------
Estimate
-------------------
tvcl 2.8138
tvv 11.005
tvvp 5.54
tvq 1.5159
tvka 2.0
Ω₁,₁ 0.10267
Ω₂,₂ 0.060776
Ω₃,₃ 1.2012
Ω₄,₄ 0.4235
Ω₅,₅ 0.24473
σ_p 0.048405
-------------------
3.3 Comparing One- versus Two-compartment models
The 2-compartment model has a much lower objective function compared to the 1-compartment. Let’s compare the estimates from the 2 models using the compare_estimates
function.
compare_estimates(; pkfit_1cmp, pkfit_2cmp)
Row | parameter | pkfit_1cmp | pkfit_2cmp |
---|---|---|---|
String | Float64? | Float64? | |
1 | tvcl | 3.1642 | 2.81378 |
2 | tvv | 13.288 | 11.0046 |
3 | tvka | 2.0 | 2.0 |
4 | Ω₁,₁ | 0.0849405 | 0.102669 |
5 | Ω₂,₂ | 0.0485682 | 0.0607757 |
6 | Ω₃,₃ | 5.58107 | 1.20115 |
7 | σ_p | 0.100928 | 0.0484049 |
8 | tvvp | missing | 5.53998 |
9 | tvq | missing | 1.51591 |
10 | Ω₄,₄ | missing | 0.423495 |
11 | Ω₅,₅ | missing | 0.244731 |
We perform a likelihood ratio test to compare the two nested models. The test statistic and the \(p\)-value clearly indicate that a 2-compartment model should be preferred.
lrtest(pkfit_1cmp, pkfit_2cmp)
Statistic: 1200.0
Degrees of freedom: 4
P-value: 0.0
We should also compare the other metrics and statistics, such ηshrinkage
, ϵshrinkage
, aic
, and bic
using the metrics_table
function.
@chain metrics_table(pkfit_2cmp) begin
leftjoin(metrics_table(pkfit_1cmp); on = :Metric, makeunique = true)
rename!(:Value => :pk2cmp, :Value_1 => :pk1cmp)
end
WARNING: using deprecated binding Distributions.MatrixReshaped in Pumas.
, use Distributions.ReshapedDistribution{2, S, D} where D<:Distributions.Distribution{Distributions.ArrayLikeVariate{1}, S} where S<:Distributions.ValueSupport instead.
Row | Metric | pk2cmp | pk1cmp |
---|---|---|---|
String | Any | Any | |
1 | Successful | true | true |
2 | Estimation Time | 16.08 | 3.115 |
3 | Subjects | 120 | 120 |
4 | Fixed Parameters | 1 | 1 |
5 | Optimized Parameters | 10 | 6 |
6 | Conc Active Observations | 1320 | 1320 |
7 | Conc Missing Observations | 0 | 0 |
8 | Total Active Observations | 1320 | 1320 |
9 | Total Missing Observations | 0 | 0 |
10 | Likelihood Approximation | Pumas.FOCE{Optim.NewtonTrustRegion{Float64}, Optim.Options{Float64, Nothing}} | Pumas.FOCE{Optim.NewtonTrustRegion{Float64}, Optim.Options{Float64, Nothing}} |
11 | LogLikelihood (LL) | 1830.63 | 1231.19 |
12 | -2LL | -3661.26 | -2462.38 |
13 | AIC | -3641.26 | -2450.38 |
14 | BIC | -3589.41 | -2419.26 |
15 | (η-shrinkage) η₁ | 0.037 | 0.016 |
16 | (η-shrinkage) η₂ | 0.047 | 0.04 |
17 | (η-shrinkage) η₃ | 0.516 | 0.733 |
18 | (ϵ-shrinkage) Conc | 0.185 | 0.105 |
19 | (η-shrinkage) η₄ | 0.287 | missing |
20 | (η-shrinkage) η₅ | 0.154 | missing |
We next generate some goodness of fit plots to compare which model is performing better. To do this, we first inspect
the diagnostics of our model fit.
= inspect(pkfit_1cmp) res_inspect_1cmp
[ Info: Calculating predictions.
[ Info: Calculating weighted residuals.
[ Info: Calculating empirical bayes.
[ Info: Evaluating individual parameters.
[ Info: Evaluating dose control parameters.
[ Info: Done.
FittedPumasModelInspection
Fitting was successful: true
Likehood approximation used for weighted residuals: FOCE
= inspect(pkfit_2cmp) res_inspect_2cmp
[ Info: Calculating predictions.
[ Info: Calculating weighted residuals.
[ Info: Calculating empirical bayes.
[ Info: Evaluating individual parameters.
[ Info: Evaluating dose control parameters.
[ Info: Done.
FittedPumasModelInspection
Fitting was successful: true
Likehood approximation used for weighted residuals: FOCE
= goodness_of_fit(res_inspect_1cmp; figure = (; fontsize = 12)) gof_1cmp
= goodness_of_fit(res_inspect_2cmp; figure = (; fontsize = 12)) gof_2cmp
These plots clearly indicate that the 2-compartment model is a better fit compared to the 1-compartment model.
We can look at selected sample of individual plots.
= subject_fits(
fig_subject_fits
res_inspect_2cmp;= true,
separate = true,
paginate = (; combinelabels = true),
facet = (; fontsize = 18),
figure = (; xlabel = "Time (hr)", ylabel = "CTMx Concentration (ng/mL)"),
axis
)1] fig_subject_fits[
There a lot of important plotting functions you can use for your standard model diagnostics. Please make sure to read the documentation for plotting. Below, we are checking the distribution of the empirical Bayes estimates.
empirical_bayes_dist(res_inspect_2cmp; zeroline_color = :red)
empirical_bayes_vs_covariates(
res_inspect_2cmp;= [:Dose],
categorical = (; size = (600, 800)),
figure )
Clearly, our guess at tvka
seems off-target. Let’s try and estimate tvka
instead of fixing it to 2
:
= fit(pk_2cmp, pkpain_noplb, init_params(pk_2cmp), FOCE()) pkfit_2cmp_unfix_ka
[ Info: Checking the initial parameter values.
[ Info: The initial negative log likelihood and its gradient are finite. Check passed.
Iter Function value Gradient norm
0 -3.200734e+02 1.272671e+03
* time: 7.891654968261719e-5
1 -8.682982e+02 1.000199e+03
* time: 0.38803911209106445
2 -1.381870e+03 5.008081e+02
* time: 0.665410041809082
3 -1.551053e+03 6.833490e+02
* time: 1.0081279277801514
4 -1.680887e+03 1.834586e+02
* time: 1.3705010414123535
5 -1.726118e+03 8.870274e+01
* time: 1.656275987625122
6 -1.761023e+03 1.162036e+02
* time: 1.9776599407196045
7 -1.786619e+03 1.114552e+02
* time: 2.3508710861206055
8 -1.863556e+03 9.914305e+01
* time: 2.7152631282806396
9 -1.882942e+03 5.342676e+01
* time: 3.027729034423828
10 -1.888020e+03 2.010181e+01
* time: 3.3563249111175537
11 -1.889832e+03 1.867263e+01
* time: 3.6934261322021484
12 -1.891649e+03 1.668512e+01
* time: 4.043851137161255
13 -1.892615e+03 1.820701e+01
* time: 4.343408107757568
14 -1.893453e+03 1.745195e+01
* time: 4.673681020736694
15 -1.894760e+03 1.850174e+01
* time: 5.008831024169922
16 -1.895647e+03 1.773939e+01
* time: 5.314917087554932
17 -1.896597e+03 1.143462e+01
* time: 5.646423101425171
18 -1.897114e+03 9.720097e+00
* time: 5.980987071990967
19 -1.897373e+03 6.054321e+00
* time: 6.32171893119812
20 -1.897498e+03 3.985954e+00
* time: 6.621455907821655
21 -1.897571e+03 4.262464e+00
* time: 6.942906141281128
22 -1.897633e+03 4.010234e+00
* time: 7.266041994094849
23 -1.897714e+03 4.805375e+00
* time: 7.606940031051636
24 -1.897802e+03 3.508706e+00
* time: 7.880497932434082
25 -1.897865e+03 3.691477e+00
* time: 8.188921928405762
26 -1.897900e+03 2.982720e+00
* time: 8.500308990478516
27 -1.897928e+03 2.563790e+00
* time: 8.768222093582153
28 -1.897968e+03 3.261485e+00
* time: 9.067466974258423
29 -1.898013e+03 3.064690e+00
* time: 9.373943090438843
30 -1.898040e+03 1.636525e+00
* time: 9.653475999832153
31 -1.898051e+03 1.439997e+00
* time: 9.955456018447876
32 -1.898057e+03 1.436504e+00
* time: 10.25761103630066
33 -1.898069e+03 1.881529e+00
* time: 10.536664009094238
34 -1.898095e+03 3.253165e+00
* time: 10.835961103439331
35 -1.898142e+03 4.257942e+00
* time: 11.144325017929077
36 -1.898199e+03 3.685241e+00
* time: 11.466082096099854
37 -1.898245e+03 2.567364e+00
* time: 11.753401041030884
38 -1.898246e+03 2.561591e+00
* time: 12.213911056518555
39 -1.898251e+03 2.530888e+00
* time: 12.654190063476562
40 -1.898298e+03 2.673696e+00
* time: 12.979318141937256
41 -1.898300e+03 2.794639e+00
* time: 13.374545097351074
42 -1.898337e+03 3.751590e+00
* time: 13.823671102523804
43 -1.898421e+03 4.878407e+00
* time: 14.114184141159058
44 -1.898433e+03 4.391719e+00
* time: 14.504771947860718
45 -1.898437e+03 4.216518e+00
* time: 14.987046957015991
46 -1.898442e+03 4.108397e+00
* time: 15.464278936386108
47 -1.898446e+03 3.934902e+00
* time: 15.946290969848633
48 -1.898449e+03 3.769838e+00
* time: 16.415061950683594
49 -1.898450e+03 3.739486e+00
* time: 16.879157066345215
50 -1.898450e+03 3.712049e+00
* time: 17.38701605796814
51 -1.898457e+03 3.623436e+00
* time: 17.82193899154663
52 -1.898471e+03 2.668312e+00
* time: 18.123503923416138
53 -1.898479e+03 2.302438e+00
* time: 18.43650794029236
54 -1.898480e+03 2.386566e-01
* time: 18.770286083221436
55 -1.898480e+03 7.802040e-01
* time: 19.096543073654175
56 -1.898480e+03 7.369786e-01
* time: 19.569113969802856
57 -1.898480e+03 5.113191e-01
* time: 19.92940402030945
58 -1.898480e+03 3.067709e-01
* time: 20.227787971496582
59 -1.898480e+03 3.076791e-01
* time: 20.535234928131104
60 -1.898480e+03 3.102066e-01
* time: 20.807610034942627
61 -1.898480e+03 3.102066e-01
* time: 21.19808292388916
62 -1.898480e+03 3.102069e-01
* time: 21.65511202812195
63 -1.898480e+03 3.102071e-01
* time: 22.308634042739868
64 -1.898480e+03 3.102074e-01
* time: 22.99835991859436
65 -1.898480e+03 3.102076e-01
* time: 23.670094966888428
66 -1.898480e+03 3.102079e-01
* time: 24.33946394920349
67 -1.898480e+03 3.102081e-01
* time: 25.008193016052246
68 -1.898480e+03 3.102081e-01
* time: 25.732465028762817
69 -1.898480e+03 3.102081e-01
* time: 26.41623592376709
70 -1.898480e+03 3.102082e-01
* time: 27.105570077896118
71 -1.898480e+03 3.102082e-01
* time: 27.772220134735107
72 -1.898480e+03 3.102082e-01
* time: 28.503803968429565
73 -1.898480e+03 3.102102e-01
* time: 29.14321804046631
74 -1.898480e+03 3.102102e-01
* time: 29.667341947555542
75 -1.898480e+03 3.102096e-01
* time: 30.139205932617188
76 -1.898480e+03 3.102096e-01
* time: 30.63081693649292
77 -1.898480e+03 3.125688e-01
* time: 31.074469089508057
78 -1.898480e+03 3.125640e-01
* time: 31.540791988372803
79 -1.898480e+03 3.125618e-01
* time: 31.98486304283142
80 -1.898480e+03 3.125615e-01
* time: 32.47225594520569
81 -1.898480e+03 3.125612e-01
* time: 32.96148490905762
82 -1.898480e+03 3.125610e-01
* time: 33.45078802108765
83 -1.898480e+03 3.125609e-01
* time: 33.992510080337524
84 -1.898480e+03 3.125604e-01
* time: 34.46971392631531
85 -1.898480e+03 3.125602e-01
* time: 34.93372201919556
86 -1.898480e+03 3.125602e-01
* time: 35.43762397766113
87 -1.898480e+03 3.125602e-01
* time: 36.12368297576904
88 -1.898480e+03 3.125602e-01
* time: 36.85410809516907
89 -1.898480e+03 3.125602e-01
* time: 37.6022469997406
90 -1.898480e+03 3.125602e-01
* time: 38.309152126312256
91 -1.898480e+03 3.125602e-01
* time: 39.029808044433594
92 -1.898480e+03 3.125602e-01
* time: 39.52189898490906
93 -1.898480e+03 3.125602e-01
* time: 40.03590703010559
94 -1.898480e+03 3.125602e-01
* time: 40.518938064575195
95 -1.898480e+03 1.387453e-01
* time: 40.87332105636597
96 -1.898480e+03 1.387453e-01
* time: 41.2874231338501
97 -1.898480e+03 1.387453e-01
* time: 41.791964054107666
98 -1.898480e+03 1.387453e-01
* time: 42.35070013999939
99 -1.898480e+03 1.387453e-01
* time: 42.72648000717163
FittedPumasModel
Successful minimization: true
Likelihood approximation: FOCE
Likelihood Optimizer: BFGS
Dynamical system type: Closed form
Log-likelihood value: 1898.4797
Number of subjects: 120
Number of parameters: Fixed Optimized
0 11
Observation records: Active Missing
Conc: 1320 0
Total: 1320 0
-------------------
Estimate
-------------------
tvcl 2.6191
tvv 11.378
tvvp 8.453
tvq 1.3164
tvka 4.8926
Ω₁,₁ 0.13243
Ω₂,₂ 0.059669
Ω₃,₃ 0.41581
Ω₄,₄ 0.080679
Ω₅,₅ 0.24996
σ_p 0.049097
-------------------
compare_estimates(; pkfit_2cmp, pkfit_2cmp_unfix_ka)
Row | parameter | pkfit_2cmp | pkfit_2cmp_unfix_ka |
---|---|---|---|
String | Float64? | Float64? | |
1 | tvcl | 2.81378 | 2.6191 |
2 | tvv | 11.0046 | 11.3784 |
3 | tvvp | 5.53998 | 8.45297 |
4 | tvq | 1.51591 | 1.31637 |
5 | tvka | 2.0 | 4.89257 |
6 | Ω₁,₁ | 0.102669 | 0.132432 |
7 | Ω₂,₂ | 0.0607757 | 0.0596693 |
8 | Ω₃,₃ | 1.20115 | 0.415811 |
9 | Ω₄,₄ | 0.423495 | 0.0806789 |
10 | Ω₅,₅ | 0.244731 | 0.249956 |
11 | σ_p | 0.0484049 | 0.0490975 |
Let’s revaluate the goodness of fits and η distribution plots.
Not much change in the general gof
plots
= inspect(pkfit_2cmp_unfix_ka) res_inspect_2cmp_unfix_ka
[ Info: Calculating predictions.
[ Info: Calculating weighted residuals.
[ Info: Calculating empirical bayes.
[ Info: Evaluating individual parameters.
[ Info: Evaluating dose control parameters.
[ Info: Done.
FittedPumasModelInspection
Fitting was successful: true
Likehood approximation used for weighted residuals: FOCE
goodness_of_fit(res_inspect_2cmp_unfix_ka; figure = (; fontsize = 12))
But you can see a huge improvement in the ηka
, (η₃
) distribution which is now centered around zero
empirical_bayes_vs_covariates(
res_inspect_2cmp_unfix_ka;= [:Dose],
categorical = [:η₃],
ebes = (; size = (600, 800)),
figure )
Finally looking at some individual plots for the same subjects as earlier:
= subject_fits(
fig_subject_fits2
res_inspect_2cmp_unfix_ka;= true,
separate = true,
paginate = (; combinelabels = true, linkyaxes = false),
facet = (; fontsize = 18),
figure = (; xlabel = "Time (hr)", ylabel = "CTMx Concentration (ng/mL)"),
axis
)6] fig_subject_fits2[
The randomly sampled individual fits don’t seem good in some individuals, but we can evaluate this via a vpc
to see how to go about.
3.4 Visual Predictive Checks (VPC)
We can now perform a vpc
to check. The default plots provide a 80% prediction interval and a 95% simulated CI (shaded area) around each of the quantiles
= vpc(
pk_vpc
pkfit_2cmp_unfix_ka,200;
= [:Conc],
observations = [:Dose],
stratify_by = EnsembleThreads(), # multi-threading
ensemblealg )
[ Info: Continuous VPC
Visual Predictive Check
Type of VPC: Continuous VPC
Simulated populations: 200
Subjects in data: 120
Stratification variable(s): [:Dose]
Confidence level: 0.95
VPC lines: quantiles ([0.1, 0.5, 0.9])
vpc_plot(
pk_2cmp,
pk_vpc;= 1,
rows = 3,
columns = (; size = (1400, 1000), fontsize = 22),
figure = (;
axis = "Time (hr)",
xlabel = "Observed/Predicted\n CTMx Concentration (ng/mL)",
ylabel
),= (; combinelabels = true),
facet )
The visual predictive check suggests that the model captures the data well across all dose levels.
4 Additional Help
If you have questions regarding this tutorial, please post them on our discourse site.