using Random
using Pumas
using PumasUtilities
using AlgebraOfGraphics
using CairoMakie
using CSV
using DataFramesMeta
using Dates
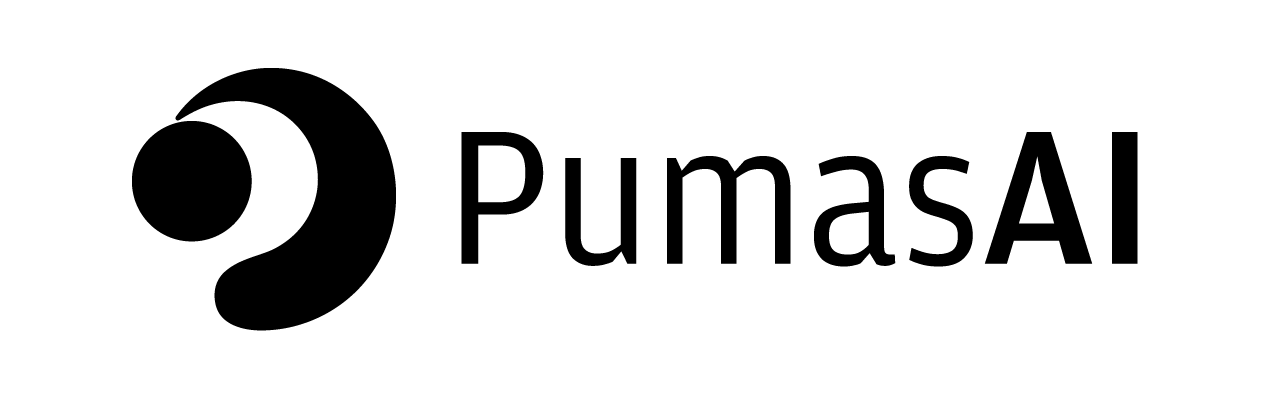
PK14 - Multi-compartment model oral dosing
1 Background
- Structural model - Two compartment linear elimination and first order absorption with lagtime
- Route of administration - Oral
- Dosage regimens - 23,158 μg single dose
- Subject - 1
2 Learning Outcome
In this model, we will simulate an oral dose to understand the disposition of a drug following lag time in absorption
3 Objectives
In this tutorial, you will learn how to build a two compartment model with lag time in oral absorption and to simulate the model for a single subject.
4 Libraries
Call the necessary libraries to get started
5 Model
In this two compartment model, we administer doses to the Depot and Central compartments.
= @model begin
pk_14 @metadata begin
= "Two Compartment Model"
desc = u"hr"
timeu end
@param begin
"""
Absorption Rate Constant (hr⁻¹)
"""
∈ RealDomain(lower = 0)
tvka """
Volume of Central Compartment (L)
"""
∈ RealDomain(lower = 0)
tvvc """
Volume of Peripheral Compartment (L)
"""
∈ RealDomain(lower = 0)
tvvp """
Clearance (L/hr)
"""
∈ RealDomain(lower = 0)
tvcl """
Intercompartmental Clearance (L/hr)
"""
∈ RealDomain(lower = 0)
tvq """
Lagtime (hr)
"""
∈ RealDomain(lower = 0)
tvlag ∈ PDiagDomain(4)
Ω """
Proportional RUV
"""
∈ RealDomain(lower = 0)
σ²_prop end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvka * exp(η[1])
Ka = tvvc * exp(η[2])
Vc = tvvp * exp(η[3])
Vp = tvcl * exp(η[4])
CL = tvq
Q end
@dosecontrol begin
= (Depot = tvlag,)
lags end
@dynamics Depots1Central1Periph1
@derived begin
= @. Central / Vc
cp """
Observed Concentrations (μg/L)
"""
~ @. Normal(cp, sqrt(cp^2 * σ²_prop))
dv end
end
PumasModel
Parameters: tvka, tvvc, tvvp, tvcl, tvq, tvlag, Ω, σ²_prop
Random effects: η
Covariates:
Dynamical system variables: Depot, Central, Peripheral
Dynamical system type: Closed form
Derived: cp, dv
Observed: cp, dv
6 Parameters
Parameters provided for simulation. Note that tv
represents the typical value for parameters.
Ka
- Absorption rate constant (hr⁻¹)Vc
- Volume of central compartment (L)Vp
- Volume of peripheral Compartmental (L)CL
- Clearance (L/hr)Q
- Intercompartmental clearance (L/hr)lag
- Absorption lag time (hr)
= (
param = 10,
tvka = 82.95,
tvvc = 54.87,
tvcl = 10.55,
tvq = 0.078,
tvlag = 107.9,
tvvp = Diagonal([0.04, 0.04, 0.04, 0.04]),
Ω = 0.0125,
σ²_prop )
7 Dosage Regimen
A single subject receives an oral dose of 23158 μg at time=0
= DosageRegimen(23158, time = 0, cmt = 1) ev1
1×10 DataFrame
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 23158.0 | 1 | 0.0 | 0 | 0.0 | 0.0 | 0 | NullRoute |
= Subject(id = 1, events = ev1) sub1
Subject
ID: 1
Events: 1
8 Simulation
Let’s simulate plasma concentration after oral dosing
Random.seed!(123)
The random effects are zero’ed out since we are simulating means
= zero_randeffs(pk_14, sub1, param) zfx
(η = [0.0, 0.0, 0.0, 0.0],)
= simobs(pk_14, sub1, param, zfx, obstimes = 0.08:0.01:25) sim_sub1
SimulatedObservations
Simulated variables: cp, dv
Time: 0.08:0.01:25.0
9 Visualization
@chain DataFrame(sim_sub1) begin
dropmissing(:cp)
data(_) *
mapping(:time => "Time (hours)", :cp => "Concentration (μg/L)") *
visual(Lines, linewidth = 4)
draw(;
= (;
axis = log10,
yscale = map(i -> 10^i, 0:0.5:2),
yticks = i -> (@. string(round(i; digits = 1))),
ytickformat = 0:5:25,
xticks
),= (; fontsize = 22),
figure
)end
10 Population simulation
= (
par = 10,
tvka = 82.95,
tvvc = 54.87,
tvcl = 10.55,
tvq = 0.078,
tvlag = 107.9,
tvvp = Diagonal([0.0152, 0.0426, 0.092, 0.0158]),
Ω = 0.03,
σ²_prop
)
= DosageRegimen(23158, time = 0, cmt = 1)
ev1 = map(i -> Subject(id = i, events = ev1), 1:68)
pop
Random.seed!(1234)
= simobs(
sim_pop
pk_14,
pop,
par,= [0.08, 0.16, 0.25, 0.5, 1, 1.5, 2, 3, 4, 6, 8, 12, 24, 25],
obstimes
)sim_plot(sim_pop, yaxis = :log)
= DataFrame(sim_pop);
df_sim
#CSV.write("pk_14.csv", df_sim)