using PumasUtilities
using Random
using Pumas
using CairoMakie
using AlgebraOfGraphics
using CSV
using DataFramesMeta
using Dates
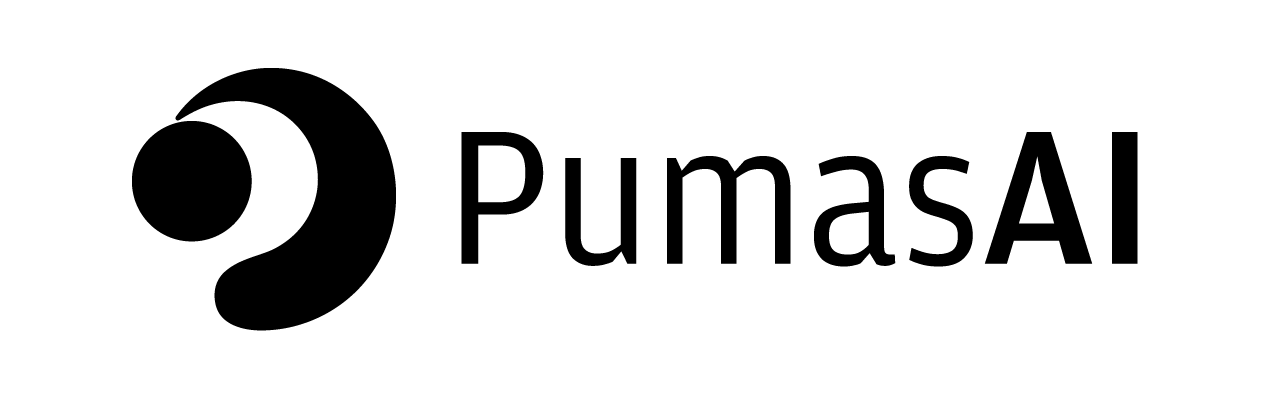
PK17 - Nonlinear Kinetics (Capacity I-IV, Flow, Hetero/Autoinduction)
1 Background
- Structural model - One compartment non-linear elimination
- Route of administration - IV infusion
- Dosage Regimen - 1800 μg rapid IV, 5484.8 μg slow IV
- Number of Subjects - 1
2 Learning Outcomes
This tutorial demonstrates simulating plasma concentration data following multiple intravenous infusions from a one compartment model taking into consideration non-linear elimination parameters.
3 Objectives
To build a one-compartment model for capacity limited kinetics, simulate the model for a single subject given multiple IV infusions, and subsequently perform a simulation for a population.
4 Libraries
Load the necessary libraries.
5 Model definition
Note the expression of the model parameters with helpful comments. The model is expressed with differential equations. Residual variability is a proportional error model.
In this one compartment model following linear elimination, multiple IV infusions are administered into the central compartment.
5.1 Linear Model
= @model begin
pk_17_ln @metadata begin
= "Linear One Compartment Model"
desc = u"minute"
timeu end
@param begin
"""
Clearance (mL/min)
"""
∈ RealDomain(lower = 0)
tvcl """
Volume of Distribution (mL)
"""
∈ RealDomain(lower = 0)
tvvc ∈ PDiagDomain(2)
Ω """
Additive RUV
"""
∈ RealDomain(lower = 0)
σ end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvcl * exp(η[1])
Cl = tvvc * exp(η[2])
Vc end
@dynamics begin
' = -(Cl / Vc) * Central
Centralend
@derived begin
= @. Central / Vc
cp """
Observed Concentration (μg/ml)
"""
~ @. Normal(cp, σ)
dv end
end
PumasModel
Parameters: tvcl, tvvc, Ω, σ
Random effects: η
Covariates:
Dynamical system variables: Central
Dynamical system type: Matrix exponential
Derived: cp, dv
Observed: cp, dv
5.2 Define Initial Estimates of Model Parameters - Linear Model
The Parameters are as given below. Note that tv
represents the typical value for parameters.
Cl
- Clearance (mL/min)Vc
- Volume of Distribution of Central Compartment (mL)Ω
- Between Subject Variabilityσ
- Residual Error
= (tvcl = 43.3, tvvc = 1380, Ω = Diagonal([0.01, 0.01]), σ = 0.00) param_ln
5.3 Michaelis Menten Model
In this one compartment model following non-linear elimination, multiple IV infusions are administered into the central compartment.
= @model begin
pk_17_mm @metadata begin
= "Michaelis Menten - One Compartment Model"
desc = u"hr"
timeu end
@param begin
"""
Maximum Metabolic Capacity (μg/min)
"""
∈ RealDomain(lower = 0)
tvvmax """
Michaelis-Menten Constant (μg/mL)
"""
∈ RealDomain(lower = 0)
tvkm """
Volume of Distribution (mL)
"""
∈ RealDomain(lower = 0)
tvvc ∈ PDiagDomain(3)
Ω """
Additive RUV
"""
∈ RealDomain(lower = 0)
σ end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvvmax * exp(η[1])
Vmax = tvkm * exp(η[2])
Km = tvvc * exp(η[3])
Vc end
@dynamics begin
' = -(Vmax / (Km + (Central / Vc))) * (Central / Vc)
Centralend
@derived begin
= @. Central / Vc
cp """
Observed Concentration (μg/ml)"
"""
~ @. Normal(cp, cp * σ)
dv end
end
PumasModel
Parameters: tvvmax, tvkm, tvvc, Ω, σ
Random effects: η
Covariates:
Dynamical system variables: Central
Dynamical system type: Nonlinear ODE
Derived: cp, dv
Observed: cp, dv
5.4 Define Initial Estimates of Model Parameters- Michaelis Menten Model
The Parameters are as given below. Note that tv
represents the typical value for parameters.
Vmax
- Maximum Metabolic Capacity (μg/min)Km
- Michaelis-Menten Constant (μg/mL)Vc
- Volume of Distribution of Central Compartment (mL)Ω
- Between Subject Variabilityσ
- Residual Error
= (
param_mm = 124.451,
tvvmax = 0.981806,
tvkm = 1350.61,
tvvc = Diagonal([0.01, 0.01, 0.01]),
Ω = 0.007,
σ )
6 Dosage Regimen
A single subject received a rapid infusion of 1800 μg over 0.5 minutes, followed by 5484.8 μg over 39.63 minutes.
= DosageRegimen([1800, 5484.8], time = [0, 0.5], cmt = [1, 1], duration = [0.5, 39.63]) DR
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 1800.0 | 1 | 0.0 | 0 | 3600.0 | 0.5 | 0 | NullRoute |
2 | 0.5 | 1 | 5484.8 | 1 | 0.0 | 0 | 138.4 | 39.63 | 0 | NullRoute |
7 Single-individual that receives the defined dose
= Subject(id = "ID:1 Linear Kinetics", events = DR) s1
Subject
ID: ID:1 Linear Kinetics
Events: 2
= Subject(id = "ID:2 Michaelis-Menten Kinetics", events = DR) s2
Subject
ID: ID:2 Michaelis-Menten Kinetics
Events: 2
8 Single-Subject Simulation
Simulate for plasma concentration for a single subject for specific observation time points after multiple IV infusion doses.
Initialize the random number generator with a seed for reproducibility of the simulation.
8.1 Linear model
Random.seed!(1234)
Define the timepoints at which concentration values will be simulated.
= simobs(pk_17_ln, s1, param_ln, obstimes = 0.0:0.01:80.35) sim_ln
SimulatedObservations
Simulated variables: cp, dv
Time: 0.0:0.01:80.35
8.2 Michaelis Menten Model
Define the timepoints at which concentration values will be simulated.
= simobs(pk_17_mm, s2, param_mm, obstimes = 0.0:0.01:80.35) sim_mm
SimulatedObservations
Simulated variables: cp, dv
Time: 0.0:0.01:80.35
9 Visualize Results
= @chain DataFrame(sim_ln) begin
plt_ln dropmissing(:cp)
data(_) *
mapping(:time => "Time (minutes)", :cp => "Concentration (μg/mL)", color = :id => "") *
visual(Lines; linewidth = 4)
end
= @chain DataFrame(sim_mm) begin
plt_mm dropmissing(:cp)
data(_) *
mapping(:time => "Time (minutes)", :cp => "Concentration (μg/mL)", color = :id => "") *
visual(Lines; linewidth = 4)
end
draw(
+ plt_mm;
plt_ln = (; xticks = 0:10:90),
axis = (; fontsize = 22),
figure = (; position = :bottom),
legend )
10 Perform a Population Simulation
We perform a population simulation with 45 participants.
This code demonstrates how to write the simulated concentrations to a comma separated file (.csv
).
= (
param = 124.451,
tvvmax = 0.981806,
tvkm = 1350.61,
tvvc = Diagonal([0.04, 0.025, 0.0025]),
Ω = 0.0573191,
σ
)
= DosageRegimen([1800, 5484.8], time = [0, 0.5], cmt = [1, 1], duration = [0.5, 39.63])
DR = map(i -> Subject(id = i, events = DR), 1:45)
pop
Random.seed!(123)
= simobs(
pop_sim
pk_17_mm,
pop,
param,= [
obstimes 0.1,
5.38,
10.33,
15.3,
20.35,
23.13,
28.15,
33.18,
38.23,
40.62,
45.25,
50.08,
60.42,
80.35,
],
)
= DataFrame(pop_sim)
pkdata_17_sim #CSV.write("pk_17_sim.csv", pkdata_17_sim);
11 Conclusion
This tutorial showed how to build a one-compartment model for IV infusion, taking into consideration non-linear elimination parameters, and perform a single subject and population simulation.