using PumasUtilities
using Random
using Pumas
using CairoMakie
using AlgebraOfGraphics
using CSV
using DataFramesMeta
using Dates
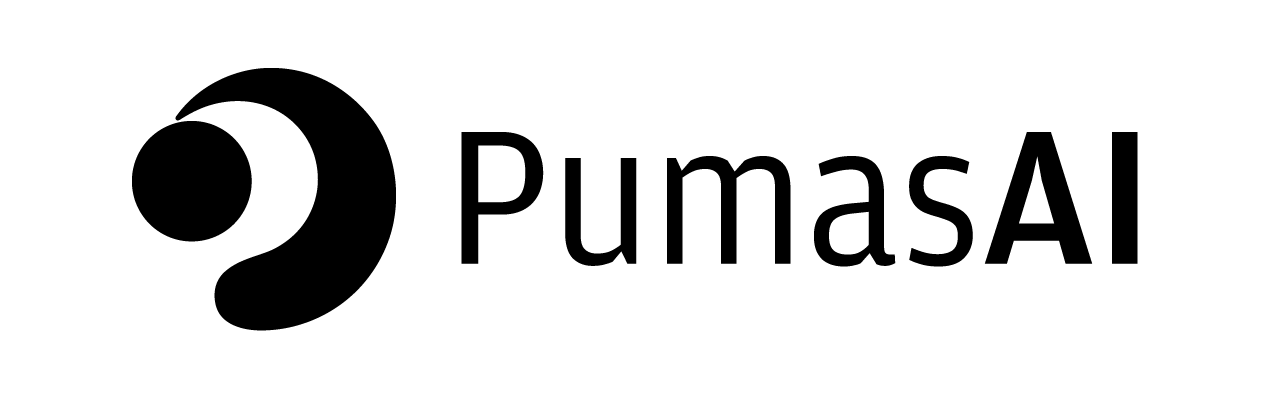
PK20 - One-compartment model with capacity limited elimination for IV bolus
1 Background
- Structural Model - One Compartment Model with nonlinear elimination.
- Route of administration - IV bolus
- Dosage Regimen - 25 mg and 100 mg
- Number of Subjects - 2
2 Learning Outcome
This exercise demonstrates simulating IV bolus with different dosage regimens and different Vmax
and Km
values with capacity limited elimination from a one compartment model.
3 Objectives
To build a one-compartment model for an IV bolus dose with capacity limited elimination, simulate the model for each subject, and subsequently perform a simulation for a population.
4 Libraries
Load the necessary libraries.
5 Model definition
Note the expression of the model parameters with helpful comments. The model is expressed with differential equations. Residual variability is a proportional error model.
= @model begin
pk_20 @metadata begin
= "One Compartment Model - Nonlinear Elimination"
desc = u"hr"
timeu end
@param begin
"""
Volume of Central Compartment (L)
"""
∈ RealDomain(lower = 0)
tvvc """
Michaelis menten Constant (μg/L)
"""
∈ RealDomain(lower = 0)
tvkm """
Maximum Rate of Metabolism (μg/hr)
"""
∈ RealDomain(lower = 0)
tvvmax ∈ PDiagDomain(3)
Ω """
Proportional RUV
"""
∈ RealDomain(lower = 0)
σ_prop end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvvc * exp(η[1])
Vc = tvkm * exp(η[2])
Km = tvvmax * exp(η[3])
Vmax end
@dynamics begin
' = -(Vmax * (Central / Vc) / (Km + (Central / Vc)))
Centralend
@derived begin
= @. Central / Vc
cp """
Observed Concentration (μg/L)
"""
~ @. Normal(cp, cp * σ_prop)
dv end
end
PumasModel
Parameters: tvvc, tvkm, tvvmax, Ω, σ_prop
Random effects: η
Covariates:
Dynamical system variables: Central
Dynamical system type: Nonlinear ODE
Derived: cp, dv
Observed: cp, dv
6 Initial Estimates of Model Parameters
The model parameters for simulation are the following. Note that tv
represents the typical value for parameters.
Km
- Michaelis menten Constant (μg/L)Vc
- Volume of Central Compartment (L),Vmax
- Maximum rate of metabolism (μg/hr),Ω
- Between Subject Variability,σ
- Residual error
A vector of model parameter values is defined.
param1
are the parameter values for Subject 1param2
are the parameter values for Subject 2
= (
param1 = 261.736,
tvkm = 36175.1,
tvvmax = 48.9892,
tvvc = Diagonal([0.01, 0.01, 0.01]),
Ω = 0.01,
σ_prop )
= (param1..., tvkm = 751.33) param2
Merge both parameters
= vcat(param1, param2) param
7 Define the Dosage Regimen and Subjects
- Subject 1 - receives an IV dose of 25 mg or 25000 μg
- Subject 2 - receives an IV dose of 100 mg or 100000 μg
= DosageRegimen(25000, time = 0, cmt = 1) ev1
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 25000.0 | 1 | 0.0 | 0 | 0.0 | 0.0 | 0 | NullRoute |
= Subject(id = 1, events = ev1, time = 0.01:0.01:2) sub1
Subject
ID: 1
Events: 1
= DosageRegimen(100000, time = 0, cmt = 1) ev2
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 100000.0 | 1 | 0.0 | 0 | 0.0 | 0.0 | 0 | NullRoute |
= Subject(id = 2, events = ev2, time = 0.01:0.01:8) sub2
Subject
ID: 2
Events: 1
= [sub1, sub2] pop
Population
Subjects: 2
Observations:
8 Single-Subject Simulation
Simulate the plasma drug concentration for the two subjects
Initialize the random number generator with a seed for reproducibility of the simulation.
Random.seed!(123)
= map(((subject, paramᵢ),) -> simobs(pk_20, subject, paramᵢ), zip(pop, param)) sim
Simulated population (Vector{<:Subject})
Simulated subjects: 2
Simulated variables: cp, dv
9 Visualize Results
@chain DataFrame(sim) begin
dropmissing(:cp)
data(_) *
mapping(
:time => "Time (hours)",
:cp => "Concentration (μg/L)";
= :id => "Subject",
color *
) visual(Lines; linewidth = 4)
draw(;
= (; fontsize = 22),
figure = (; xticks = 0:1:8),
axis = (; position = :bottom),
legend
)end
10 Population Simulation
We perform a population simulation with 45 participants.
This code demonstrates how to write the simulated concentrations to a comma separated file (.csv
).
= (
par1 = 261.736,
tvkm = 36175.1,
tvvmax = 48.9892,
tvvc = Diagonal([0.09, 0.04, 0.0225]),
Ω = 0.0927233,
σ_prop
)
= (
par2 = 751.33,
tvkm = 36175.1,
tvvmax = 48.9892,
tvvc = Diagonal([0.04, 0.09, 0.0225]),
Ω = 0.0628022,
σ_prop
)
## Subject 1
= DosageRegimen(25000, time = 0, cmt = 1)
ev1 = map(i -> Subject(id = i, events = ev1), 1:45)
pop1
Random.seed!(1234)
= simobs(pk_20, pop1, par1, obstimes = [0.08, 0.25, 0.5, 0.75, 1, 1.5, 2])
sim_pop1 sim_plot(sim_pop1, yaxis = :log)
= DataFrame(sim_pop1)
df1_pop
## Subject 2
= DosageRegimen(100000, time = 0, cmt = 1)
ev2 = map(i -> Subject(id = 1, events = ev2), 1:45)
pop2
Random.seed!(1234)
= simobs(pk_20, pop2, par2, obstimes = [0.08, 0.25, 0.5, 0.75, 1, 1.5, 2, 4, 6, 8])
sim_pop2 sim_plot(sim_pop2, yaxis = :log)
= DataFrame(sim_pop2)
df2_pop
= vcat(df1_pop, df2_pop)
pkdata_20_sim
#CSV.write("pk_20_sim.csv", pkdata_20_sim)
11 Conclusion
This tutorial showed how to build a one compartment model for an IV bolus dose with capacity limited elimination and perform a single subject and population simulation.