using Random
using Pumas
using PumasUtilities
using AlgebraOfGraphics
using CairoMakie
using CSV
using DataFramesMeta
using Dates
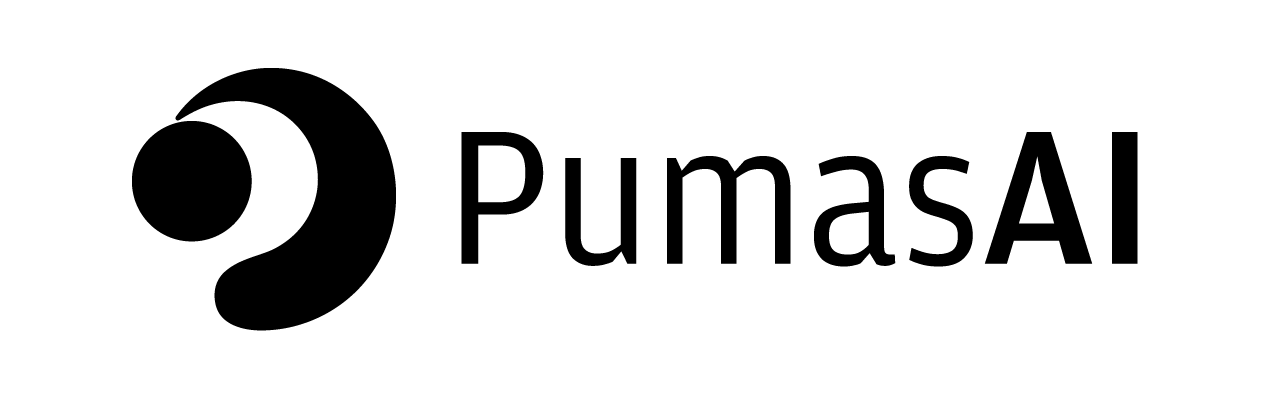
PK22 - Non-linear Kinetics - Autoinduction
1 Background
- Structural model - Two compartment Model for drug and one compartment model for enzyme
- Route of administration - IV Infusion
- Dosage Regimen - 120 mg of IV infusion at a constant rate of 1 hour followed by 9 more doses of 40 mg for 30 minutes duration at the interval of 8 hours
- Number of Subjects - 1
2 Learning Outcome
This model gives an understanding of drug pharmacokinetics and enzyme autoinduction simultaneously after repeated IV infusion.
3 Objectives
In this tutorial, you will learn how to build a two compartment model for a drug and a one compartment model for an enzyme and simulate from the model.
4 Libraries
Call the necessary libraries to get started.
5 Model
In this two compartment model, we administer repeated doses of IV infusions to a single subject.
= @model begin
pk_22 @metadata begin
= "Autoinduction Model"
desc = u"hr"
timeu end
@param begin
"""
Clearance at Steady State (L/hr)
"""
∈ RealDomain(lower = 0)
tvcls """
Central Volume of Distribution (L)
"""
∈ RealDomain(lower = 0)
tvvc """
Peripheral Volume of Distribution (L)
"""
∈ RealDomain(lower = 0)
tvvp """
Distribution Clearance (L/hr)
"""
∈ RealDomain(lower = 0)
tvq """
Input Rate Constant for Enzyme (hr⁻¹)
"""
∈ RealDomain(lower = 0)
tvkin """
Output Rate Constant for Enzyme (hr⁻¹)
"""
∈ RealDomain(lower = 0)
tvkout """
Initial Enzyme Concentration
"""
∈ RealDomain(lower = 0)
tvE0 ∈ PDiagDomain(7)
Ω """
Proportional RUV
"""
∈ RealDomain(lower = 0)
σ²_prop end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvcls * exp(η[1])
Cls = tvvc * exp(η[2])
Vc = tvvp * exp(η[3])
Vp = tvq * exp(η[4])
Q = tvkin * exp(η[5])
Kin = tvkout * exp(η[6])
Kout = tvE0 * exp(η[7])
E0 end
@init begin
= (Kin / Kout) + E0
Enzyme end
@dynamics begin
' =
Central-(Cls / Vc) * Central * Enzyme + (Q / Vp) * Peripheral - (Q / Vc) * Central
' = (Q / Vc) * Central - (Q / Vp) * Peripheral
Peripheral' = Kin * (E0 + Central / Vc) - Kout * Enzyme
Enzymeend
@derived begin
= @. Central / Vc
cp """
Observed Concentration (mcg/L))
"""
~ @. Normal(cp, sqrt(cp^2 * σ²_prop))
dv end
end
PumasModel
Parameters: tvcls, tvvc, tvvp, tvq, tvkin, tvkout, tvE0, Ω, σ²_prop
Random effects: η
Covariates:
Dynamical system variables: Central, Peripheral, Enzyme
Dynamical system type: Nonlinear ODE
Derived: cp, dv
Observed: cp, dv
6 Parameters
Parameters are provided for the simulation. Note that tv
represents the typical value for parameters.
tvcls
- Typical Value Clearance at Steady State (L/hr)tvvc
- Typical Value Central Volume of Distribution (L)tvvp
- Typical Value Peripheral Volume of Distribution (L)tvq
- Typical Value Distribution Clearance (L/hr)tvkin
- Input Rate Constant for Enzyme (hr⁻¹)tvkout
- Output Rate Constant for Enzyme (hr⁻¹)tvE0
- Initial Enzyme ConcentrationΩ
- Between Subject Variabilityσ
- Residual errors
= (
param = 0.04,
tvcls = 150.453,
tvvc = 54.0607,
tvvp = 97.8034,
tvq = 0.0238896,
tvkin = 0.0238896,
tvkout = 132.864,
tvE0 = Diagonal([0.04, 0.04, 0.04, 0.04, 0.04, 0.04, 0.04]),
Ω = 0.005,
σ²_prop )
7 Dosage Regimen
Dosage Regimen - 120 mg of IV infusion at a constant rate of 1 hour, followed by 9 more doses of 40 mg for 30 minutes duration at an interval of 8 hours
= DosageRegimen(
ev1 120000, 40000],
[= [0, 8],
time = [1, 0.5],
duration = [1, 1],
cmt = [0, 8],
addl = [0, 8],
ii )
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 120000.0 | 1 | 0.0 | 0 | 120000.0 | 1.0 | 0 | NullRoute |
2 | 8.0 | 1 | 40000.0 | 1 | 8.0 | 8 | 80000.0 | 0.5 | 0 | NullRoute |
= Subject(id = 1, events = ev1, observations = (cp = nothing,)) sub1
Subject
ID: 1
Events: 10
Observations: cp: (n=0)
8 Simulation
Let’s simulate for plasma concentration with the specific observation time points after IV.
Random.seed!(123)
The random effects are zero’ed out since we are simulating means
= zero_randeffs(pk_22, sub1, param) zfx
(η = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0],)
= simobs(pk_22, sub1, param, zfx, obstimes = 0.00:0.01:96) sim_sub1
SimulatedObservations
Simulated variables: cp, dv
Time: 0.0:0.01:96.0
9 Visualization
@chain DataFrame(sim_sub1) begin
dropmissing(:cp)
data(_) *
mapping(:time => "Time (hour)", :cp => "PK22 Concentrations (μg/mL)") *
visual(Lines, linewidth = 4)
draw(; axis = (; xticks = 0:10:100), figure = (; fontsize = 22))
end
10 Population simulation
= (
par = 0.04,
tvcls = 150.453,
tvvc = 54.0607,
tvvp = 97.8034,
tvq = 0.0238896,
tvkin = 0.0238896,
tvkout = 132.864,
tvE0 = Diagonal([0.0425, 0.0312, 0.0264, 0.0429, 0.0110, 0.0156, 0.0289]),
Ω = 0.00140536,
σ²_prop
)= DosageRegimen(
ev1 120000, 40000],
[= [0, 8],
time = [1, 0.5],
duration = [1, 1],
cmt = [0, 8],
addl = [0, 8],
ii
)= map(i -> Subject(id = i, events = ev1), 1:50)
pop Random.seed!(1234)
= simobs(
pop_sim
pk_22,
pop,
par,= [
obstimes 0.25,
0.5,
1,
1.25,
1.5,
3,
5,
7,
7.75,
8.5,
15.75,
16.5,
23.99,
24.5,
31.75,
32.5,
39.75,
40.5,
47.99,
48.5,
55.75,
56.5,
63.75,
64.5,
72,
72.25,
72.5,
72.75,
73,
73.5,
74.5,
76.5,
78.5,
80.5,
84.5,
90.5,
96,
],
);
= DataFrame(pop_sim)
df_sim #CSV.write("pk_22.csv", df_sim)