using Random
using Pumas
using PumasUtilities
using CairoMakie
using AlgebraOfGraphics
using DataFramesMeta
using CSV
using Dates
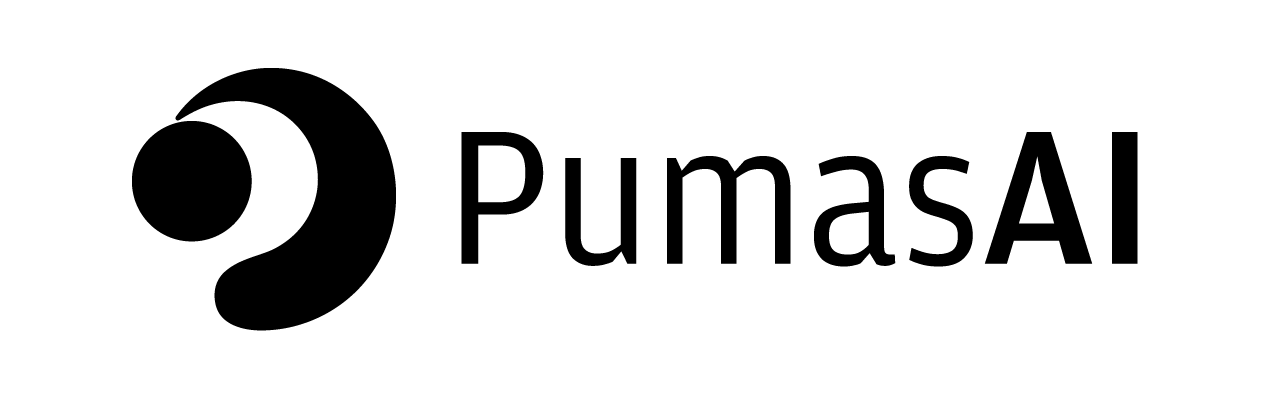
PK41 - Multiple Intravenous Infusions: NCA vs Regression
1 Background
- Structural Model - One compartment model with non-linear elimination.
- Route of administration - IV infusion
- Dosage Regimen - 310 μg, 520 μg, and 780 μg
- Number of Subjects - 3
2 Learning Outcome
This is a one compartment model with capacity limited elimination. The concentration time profile was obtained for three subjects administered with three different dosage regimens.
3 Objectives
In this tutorial, you will learn how to build a one compartment model with non-linear elimination.
4 Libraries
Call the necessary libraries to get started.
5 Model
The following model describes the parameters and the differential equation for a one-compartment model with capacity limited elimination
= @model begin
pk_41 @metadata begin
= "One Compartment Model"
desc = u"hr"
timeu end
@param begin
"""
Maximum Metabolic Rate (μg/kg/hr)
"""
∈ RealDomain(lower = 0)
tvvmax """
Michaelis Menten Constant (μg/kg/L)
"""
∈ RealDomain(lower = 0)
tvkm """
Volume of Central Compartment (L/kg)
"""
∈ RealDomain(lower = 0)
tvvc ∈ PDiagDomain(3)
Ω """
Proportional RUV
"""
∈ RealDomain(lower = 0)
σ²_prop end
@random begin
~ MvNormal(Ω)
η end
@pre begin
= tvvmax * exp(η[1])
Vmax = tvkm * exp(η[2])
Km = tvvc * exp(η[3])
Vc end
@dynamics begin
' = -(Vmax * (Central / Vc) / (Km + (Central / Vc)))
Centralend
@vars begin
= Central / Vc
cp end
@derived begin
"""
Observed Concentrations (μg/L)
"""
~ @. Normal(cp, sqrt(cp^2 * σ²_prop))
dv end
@observed begin
:= @nca cp
nca = NCA.cl(nca)
cl end
end
PumasModel
Parameters: tvvmax, tvkm, tvvc, Ω, σ²_prop
Random effects: η
Covariates:
Dynamical system variables: Central
Dynamical system type: Nonlinear ODE
Derived: dv, cp
Observed: dv, cl, cp
6 Parameters
The parameters are as given below. Note that tv
represents the typical value for parameters.
Vmax
- Maximum Metabolic Rate (μg/kg/hr)Km
- Michaelis Menten Constant (μg/kg/L)Vc
- Volume of Central compartment (L/kg)
= (
param = 180.311,
tvvmax = 79.8382,
tvkm = 1.80036,
tvvc = Diagonal([0.04, 0.04, 0.04]),
Ω = 0.015,
σ²_prop )
7 Dosage Regimen
All subjects receive an IV infusion over 5 hours with the following doses:
- Subject 1 receives a dose of 310 μg
- Subject 2 receives a dose of 520 μg
- Subject 3 receives a dose of 780 μg
= [310, 520, 780] dose
= [62, 104, 156] rate_ind
= ["310 μg.kg-1", "520 μg.kg-1", "780 μg.kg-1"] ids
ev(dose, rate) = DosageRegimen(dose; cmt = 1, time = 0, rate, route = NCA.IVInfusion)
ev (generic function with 1 method)
=
pop3_sub map((id, dose, rate) -> Subject(; id, events = ev(dose, rate)), ids, dose, rate_ind)
Population
Subjects: 3
Observations:
8 Simulation
Simulate the plasma concentration of the drug for all the subjects
Random.seed!(123)
The random effects are zero’ed out since we are simulating a single subject
= zero_randeffs(pk_41, pop3_sub, param) zfx
3-element Vector{@NamedTuple{η::Vector{Float64}}}:
(η = [0.0, 0.0, 0.0],)
(η = [0.0, 0.0, 0.0],)
(η = [0.0, 0.0, 0.0],)
= 0.1:0.01:10
time_values = simobs(pk_41, pop3_sub, param, zfx, obstimes = time_values) sim_pop3_sub
Simulated population (Vector{<:Subject})
Simulated subjects: 3
Simulated variables: dv, cp
9 Visualization
We will build a DataFrame
to facilitate plotting
= DataFrame(sim_pop3_sub; include_events = false) df_plot
=
layer data(df_plot) *
mapping(:time => "Time (hours)", :cp => "Concentration (μg/L)", color = :id => "Dose") *
visual(Lines; linewidth = 4)
draw(
layer;= (; fontsize = 22),
figure = (;
axis = 0:10,
xticks = log10,
yscale = i -> (@. string(round(i; digits = 1))),
ytickformat
),= (; position = :bottom),
legend )
=
layer data(unique(df_plot, [:id, :cl])) *
mapping(:id => nonnumeric => "Dose", :cl => "Cl (L/hr/kg)") *
visual(ScatterLines, linewidth = 4, markersize = 22)
draw(layer; axis = (; yticks = 0.8:0.2:1.8,), figure = (; fontsize = 22))
10 Simulating a Population
= (
par = 180.311,
tvvmax = 79.8382,
tvkm = 1.80036,
tvvc = Diagonal([0.0462, 0.0628, 0.0156]),
Ω = 0.0234,
σ²_prop
)
= 1:60
ids = repeat([310, 520, 780]; inner = 20)
doses = repeat([62, 104, 156]; inner = 20)
rates = map(ids, doses, rates) do id, dose, rate
pop = DosageRegimen(dose; cmt = 1, time = 0, rate, route = NCA.IVInfusion)
events return Subject(; id, events)
end
Random.seed!(1234)
= simobs(pk_41, pop, par, obstimes = [0, 0.1, 2, 5, 6, 8, 10])
sim_pop
= select(DataFrame(sim_pop; include_events = false), [:id, :time, :cp, :cl])
df_sim
write("pk_41.csv", df_sim) CSV.
"pk_41.csv"