using PumasUtilities
using Random
using Pumas
using CairoMakie
using AlgebraOfGraphics
using DataFramesMeta
using CSV
using Dates
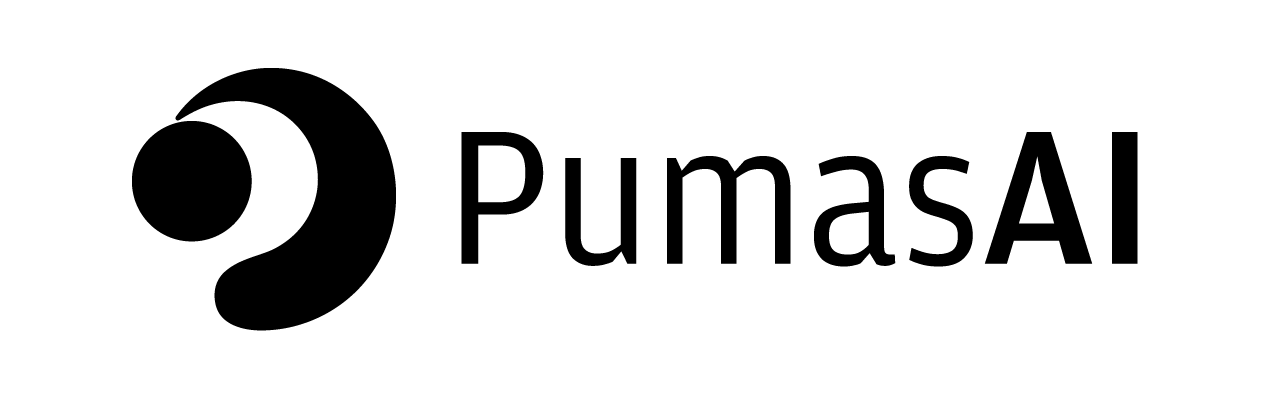
PK44 (Part 1) - Estimation of inhibitory constant Ki
1 Background
- Structural model - Estimation of inhibitory rate constant in competitive enzyme inhibition model
- Number of subjects - 1
- Number of compounds - 1
2 Learning Outcome
This exercise demonstrates how to build a competitive inhibitory model and understand the relationship between rate of metabolite formation and concentration.
3 Objectives
To build a competitive inhibitory model and simulate the model for a single subject.
4 Libraries
Load the necessary libraries
5 Model definition
Note the expression of the model parameters with helpful comments. The model is expressed with differential equations. Residual variability is an additive error model.
= @model begin
pk_44_cim @metadata begin
= "Competitive Inhibitory Model"
desc = u"minute"
timeu end
@param begin
"""
Maximum metabolic rate (μM*gm/min)
"""
∈ RealDomain(lower = 0)
tvvmax """
Michaelis-Mentons constant (μmol/L)
"""
∈ RealDomain(lower = 0)
tvkm """
Inhibitory constant (μmol/L)
"""
∈ RealDomain(lower = 0)
tvki ∈ PDiagDomain(2)
Ω """
Additive RUV
"""
∈ RealDomain(lower = 0)
σ_add end
@random begin
~ MvNormal(Ω)
η end
@covariates conc I
@pre begin
= tvvmax * exp(η[1])
Vmax = tvkm * exp(η[2])
Km = tvki
Ki = conc
_conc = I
_I end
@derived begin
## Competitive Inhibiton Model
= @. Vmax * _conc / ((Km * (1 + (_I / Ki)) + _conc))
rate_cim """
Metabolic rate (nmol/min/mg)
"""
~ @. Normal(rate_cim, σ_add)
dv_rate_cim end
end
PumasModel
Parameters: tvvmax, tvkm, tvki, Ω, σ_add
Random effects: η
Covariates: conc, I
Dynamical system variables:
Dynamical system type: No dynamical model
Derived: rate_cim, dv_rate_cim
Observed: rate_cim, dv_rate_cim
6 Initial Estimates of Model Parameters
The model parameters for simulation are the following. Note that tv
represents the typical value for parameters.
Vmax
- Maximum metabolic rate (μM*gm_protein/min)Km
- Michaelis-Mentons constant (μmol/L)Ki
- Inhibitory constant (μmol/L)I
- Inhibitor concentration/Exposure
= (
param = 99.8039,
tvvmax = 11.3192,
tvkm = 5.05605,
tvki = Diagonal([0.01, 0.01]),
Ω = 1.256,
σ_add )
7 Creating a Dataset
The time, concentration data and exposure (I) will be used to estimate the rate of metabolite concentration.
= map(
df_sub1 -> DataFrame(id = i, time = 1:1:1000, dv_rate_cim = missing, conc = 1:1:1000, I = 0),
i 1:6,
)= vcat(DataFrame.(df_sub1)...)
df :I] = ifelse.(df.id .== 2, 10, df.I)
df[!, :I] = ifelse.(df.id .== 3, 25, df.I)
df[!, :I] = ifelse.(df.id .== 4, 50, df.I)
df[!, :I] = ifelse.(df.id .== 5, 75, df.I)
df[!, :I] = ifelse.(df.id .== 6, 100, df.I)
df[!, = df
df_sub1 = read_pumas(
sub1
df_sub1,= [:dv_rate_cim],
observations = [:conc, :I],
covariates = false,
event_data )
Population
Subjects: 6
Covariates: conc, I
Observations: dv_rate_cim
8 Single-Subject Simulation
Simulate the rate of metabolite formation
Initialize the random number generator with a seed for reproducibility of the simulation.
Random.seed!(123)
= simobs(pk_44_cim, sub1, param) sim_sub1
Simulated population (Vector{<:Subject})
Simulated subjects: 6
Simulated variables: rate_cim, dv_rate_cim
= DataFrame(sim_sub1)
pkdata_44_1 first(pkdata_44_1, 5)
Row | id | time | rate_cim | dv_rate_cim | evid | conc | I | Vmax | Km | Ki | _conc | _I | η_1 | η_2 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
String | Float64 | Float64? | Float64? | Int64 | Int64? | Int64? | Float64? | Float64? | Float64? | Int64? | Int64? | Float64 | Float64 | |
1 | 1 | 1.0 | 9.28393 | 9.56195 | 0 | 1 | 0 | 125.433 | 12.5108 | 5.05605 | 1 | 0 | 0.228566 | 0.100091 |
2 | 1 | 2.0 | 17.2883 | 17.9765 | 0 | 2 | 0 | 125.433 | 12.5108 | 5.05605 | 2 | 0 | 0.228566 | 0.100091 |
3 | 1 | 3.0 | 24.2605 | 25.6057 | 0 | 3 | 0 | 125.433 | 12.5108 | 5.05605 | 3 | 0 | 0.228566 | 0.100091 |
4 | 1 | 4.0 | 30.3882 | 30.4912 | 0 | 4 | 0 | 125.433 | 12.5108 | 5.05605 | 4 | 0 | 0.228566 | 0.100091 |
5 | 1 | 5.0 | 35.816 | 34.9926 | 0 | 5 | 0 | 125.433 | 12.5108 | 5.05605 | 5 | 0 | 0.228566 | 0.100091 |
9 Visualize Results
@chain pkdata_44_1 begin
@rsubset :conc ∈ [1, 5, 10, 15, 26, 104, 251, 502, 1000]
data(_) *
mapping(
:conc => "Concentration (μM)",
:rate_cim => "Metabolic rate (nmol/min/mg protein)",
= :I => nonnumeric => "Exposure",
color *
) visual(ScatterLines, linewidth = 4, markersize = 12)
draw(; figure = (; fontsize = 22), axis = (; xticks = 0:100:1000, yticks = 0:10:100))
end
@chain pkdata_44_1 begin
@rsubset :conc ∈ [1, 5, 10, 15, 26, 104, 251, 502, 1000]
data(_) *
mapping(
:conc => "log Concentration (μM)",
:rate_cim => "Metabolic rate (nmol/min/mg protein)",
= :I => nonnumeric => "Exposure",
color *
) visual(ScatterLines, linewidth = 4, markersize = 12)
draw(;
= (; fontsize = 22),
figure = (;
axis = log10,
xscale = i -> (@. string(round(i; digits = 1))),
xtickformat = 0:10:100,
yticks
),
)end
10 Conclusion
This tutorial showed how to build a competitive inhibitory model and perform a rate of metabolite formation versus concentration simulation.