using Random
using Pumas
using PumasUtilities
using CairoMakie
Random.seed!(1234) # Set random seed for reproducibility
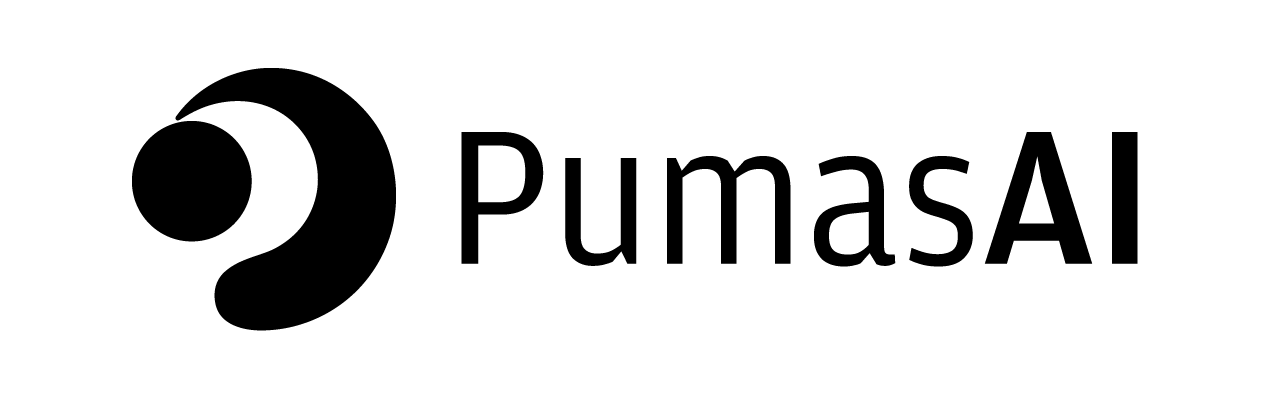
Absorption Models
1 Introduction
Absorption modeling is an integral part of pharmacokinetic modeling for drugs delivered by any route of administration other than intravenous (IV) (e.g. oral, subcutaneous, intramuscular, transdermal, nasal, etc.). In the simplest case, drugs administered orally may undergo first-order absorption, whereby the absorption from the depot compartment (i.e. gut) to the central compartment (i.e. plasma) is a first-order process. However, many more complex situations arise in practice, some of which will becovered in this tutorial.
We will cover the following absorption models:
- First-order
- Zero-order
- Parallel zero-order and first-order
- Two parallel first-order processes
- Weibull-type absorption
- Absorption through a sequence of transit compartments (Erlang absorption)
2 Generating the Population
We begin by simulating a population of 10 subjects to use for illustration purposes in this tutorial. For simplicity, we will use a 1-compartment distribution system with additional absorption compartment(s) as required. Note that in these examples we are simulating without residual error (it could be added, but isn’t necessary here). Hence, we use the @observed
block that is designed to capture post dynamics outputs.
= DosageRegimen(100; time = 0) dose1
Row | time | cmt | amt | evid | ii | addl | rate | duration | ss | route |
---|---|---|---|---|---|---|---|---|---|---|
Float64 | Int64 | Float64 | Int8 | Float64 | Int64 | Float64 | Float64 | Int8 | NCA.Route | |
1 | 0.0 | 1 | 100.0 | 1 | 0.0 | 0 | 0.0 | 0.0 | 0 | NullRoute |
choose_covariates() = (; wt = rand(55:80), dose = 100)
choose_covariates (generic function with 1 method)
= map(
subj_with_covariates1 -> Subject(;
i = i,
id = dose1,
events = choose_covariates(),
covariates = (; conc = nothing),
observations
),1:10,
)
Population
Subjects: 10
Covariates: wt, dose
Observations: conc
For a more in-depth discussion of simulating data with Pumas, please see the tutorial generating and simulation populations.
3 First-Order Absorption
Note that we have written the model using differential equations, but we could equally have specified an “analytical” (closed-form) solution using this @dynamics
block instead (see documentation on analytical solutions):
@dynamics Depots1Central1
Here is the Pumas code for a 1-compartment model with first-order absorption, diagonal random-effects structure, and allometric scaling on clearance and volume:
= @model begin
foabs
@param begin
∈ RealDomain(; lower = 0)
tvcl ∈ RealDomain(; lower = 0)
tvvc ∈ RealDomain(; lower = 0)
tvka ∈ PDiagDomain(3)
Ω end
@random begin
~ MvNormal(Ω)
η end
@covariates wt
@pre begin
= tvcl * (wt / 70)^0.75 * exp(η[1])
CL = tvvc * (wt / 70) * exp(η[2])
Vc = tvka * exp(η[3])
Ka end
@dynamics begin
' = -Ka * Depot
Depot' = Ka * Depot - (CL / Vc) * Central
Centralend
@observed begin
= @. Central / Vc
conc end
end
PumasModel
Parameters: tvcl, tvvc, tvka, Ω
Random effects: η
Covariates: wt
Dynamical system variables: Depot, Central
Dynamical system type: Matrix exponential
Derived:
Observed: conc
= (; tvcl = 5, tvvc = 20, tvka = 1, Ω = Diagonal([0.04, 0.04, 0.04]))
param1 = simobs(foabs, subj_with_covariates1, param1; obstimes = 0:0.1:24) sims1
Simulated population (Vector{<:Subject})
Simulated subjects: 10
Simulated variables: conc
sim_plot(
sims1;= [:conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Predicted Concentration (ng/mL)",
ylabel = "First-order absorption",
title
), )
4 Zero-Order Absorption
Notice that there is no depot compartment, and that instead the absorption takes place in the @dosecontrol
block by specifying a duration
for the zero-order process. In order for this to work, we need to set the rate
data item in DosageRegimen
to the value -2
; this is a clue to the Pumas engine that the rate
should be derived from the duration
and amt
.
Zero-order absorption is less common than first-order absorption. It is essentially like an IV infusion, but where the duration of the infusion is an estimated parameter rather than a known quantity. Here is the Pumas code for the same 1-compartment model but with zero-order absorption:
= @model begin
zoabs @param begin
∈ RealDomain(; lower = 0)
tvcl ∈ RealDomain(; lower = 0)
tvvc ∈ RealDomain(; lower = 0)
tvdur ∈ PDiagDomain(3)
Ω end
@random begin
~ MvNormal(Ω)
η end
@covariates wt
@pre begin
= tvcl * (wt / 70)^0.75 * exp(η[1])
CL = tvvc * (wt / 70) * exp(η[2])
Vc end
@dosecontrol begin
= (; Central = tvdur * exp(η[3]))
duration end
@dynamics begin
' = -(CL / Vc) * Central
Centralend
@observed begin
= @. Central / Vc
conc end
end
PumasModel
Parameters: tvcl, tvvc, tvdur, Ω
Random effects: η
Covariates: wt
Dynamical system variables: Central
Dynamical system type: Matrix exponential
Derived:
Observed: conc
= (; tvcl = 0.792, tvvc = 13.7, tvdur = 5.0, Ω = Diagonal([0.04, 0.04, 0.04]))
param2 = DosageRegimen(
dose2 100;
= 0,
time = -2, # Note: rate must be -2 for duration modeling
rate
)= map(
subj_with_covariates2 -> Subject(;
i = i,
id = dose2,
events = choose_covariates(),
covariates = (; conc = nothing),
observations
),1:10,
)= simobs(zoabs, subj_with_covariates2, param2; obstimes = 0:0.1:48) sims2
Simulated population (Vector{<:Subject})
Simulated subjects: 10
Simulated variables: conc
sim_plot(
zoabs,
sims2;= [:conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Predicted Concentration (ng/mL)",
ylabel = "Zero-order absorption",
title
), )
5 Parallel Zero-Order and First-Order Absorption
This is a more complex absorption model, with both a zero-order process and a first-order process operating simultaneously. Essentially, the total dose is split into two parts, one of which undergoes first-order absorption, and the other zero-order absorption (how this splitting is typically accomplished from a technical perspective is to treat each actual dose as two virtual doses with different bioavailable fractions, as in the example below). Furthermore, we will assume that the first-order process is subject to a lag (i.e., the absorption begins after a certain time delay, which is a model parameter).
= @model begin
zofo_paral_abs
@param begin
∈ RealDomain(; lower = 0)
tvcl ∈ RealDomain(; lower = 0)
tvvc ∈ RealDomain(; lower = 0)
tvka ∈ RealDomain(; lower = 0)
tvdur ∈ RealDomain(; lower = 0)
tvbio ∈ RealDomain(; lower = 0)
tvlag ∈ PDiagDomain(2)
Ω end
@random begin
~ MvNormal(Ω)
η end
@covariates wt
@pre begin
= tvcl * (wt / 70)^0.75 * exp(η[1])
CL = tvvc * (wt / 70) * exp(η[2])
Vc = tvka
Ka end
@dosecontrol begin
= (; Central = tvdur)
duration = (; Depot = tvbio, Central = 1 - tvbio)
bioav = (; Depot = tvlag)
lags end
@dynamics begin
' = -Ka * Depot
Depot' = Ka * Depot - (CL / Vc) * Central
Centralend
@observed begin
= @. Central / Vc
conc end
end
PumasModel
Parameters: tvcl, tvvc, tvka, tvdur, tvbio, tvlag, Ω
Random effects: η
Covariates: wt
Dynamical system variables: Depot, Central
Dynamical system type: Matrix exponential
Derived:
Observed: conc
With these parameter values, the first-order process starts 1 hour after administration, while the zero-order process lasts 2 hours. Hence, there is one hour where they overlap.
= (;
param3 = 5,
tvcl = 50,
tvvc = 1.2,
tvka = 2,
tvdur = 0.5,
tvbio = 1,
tvlag = Diagonal([0.04, 0.04]),
Ω
)= DosageRegimen(
dose_zo 100;
= 0,
time = 2,
cmt = -2, # Note: rate must be -2 for duration modeling
rate = 1,
evid
)= DosageRegimen(100; time = 0, cmt = 1, rate = 0, evid = 1)
dose_fo = DosageRegimen(dose_fo, dose_zo) # Actual dose is made up of 2 virtual doses
dose3 = map(
subj_with_covariates3 -> Subject(;
i = i,
id = dose3,
events = choose_covariates(),
covariates = (; conc = nothing),
observations
),1:10,
)= simobs(zofo_paral_abs, subj_with_covariates3, param3; obstimes = 0:0.1:24) sims3
Simulated population (Vector{<:Subject})
Simulated subjects: 10
Simulated variables: conc
sim_plot(
sims3;= [:conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Predicted Concentration (ng/mL)",
ylabel = "Zero- and first-order parallel absorption",
title
), )
6 Absorption by Two Parallel First-Order Processes
This is similar to the example above, except that both parallel processes are first-order rather than one being zero-order. We will refer to the two processes as “immediate release” (IR) and “slow release” (SR); only the SR process is subject to a lag. The Pumas code is as follows:
= @model begin
two_parallel_foabs
@param begin
∈ RealDomain(; lower = 0)
tvcl ∈ RealDomain(; lower = 0)
tvvc ∈ RealDomain(; lower = 0)
tvka1 ∈ RealDomain(; lower = 0)
tvka2 ∈ RealDomain(; lower = 0)
tvlag ∈ RealDomain(; lower = 0)
tvbio ∈ PDiagDomain(6)
Ω end
@random begin
~ MvNormal(Ω)
η end
@covariates wt
@pre begin
= tvcl * (wt / 70)^0.75 * exp(η[1])
CL = tvvc * (wt / 70) * exp(η[2])
Vc = tvka1 * exp(η[3])
Ka1 = tvka2 * exp(η[4])
Ka2 end
@dosecontrol begin
= (; SR = tvlag * exp(η[5]))
lags = (; IR = tvbio * exp(η[6]), SR = (1 - tvbio) * exp(η[6]))
bioav end
@dynamics begin
' = -Ka1 * IR
IR' = -Ka2 * SR
SR' = Ka1 * IR + Ka2 * SR - Central * CL / Vc
Centralend
@observed begin
= @. Central / Vc
conc end
end
PumasModel
Parameters: tvcl, tvvc, tvka1, tvka2, tvlag, tvbio, Ω
Random effects: η
Covariates: wt
Dynamical system variables: IR, SR, Central
Dynamical system type: Matrix exponential
Derived:
Observed: conc
= (;
param4 = 5,
tvcl = 50,
tvvc = 0.8,
tvka1 = 0.6,
tvka2 = 5,
tvlag = 0.5,
tvbio = Diagonal([0.04, 0.04, 0.36, 0.36, 0.04, 0.04]),
Ω
)= DosageRegimen(100; cmt = 1, time = 0)
dose_fo1 = DosageRegimen(100; cmt = 2, time = 0)
dose_fo2 = DosageRegimen(dose_fo1, dose_fo2) # Actual dose is made up of 2 virtual doses
dose4 = map(
subj_with_covariates4 -> Subject(;
i = i,
id = dose4,
events = choose_covariates(),
covariates = (; conc = nothing),
observations
),1:10,
)= simobs(two_parallel_foabs, subj_with_covariates4, param4; obstimes = 0:0.1:48) sims4
Simulated population (Vector{<:Subject})
Simulated subjects: 10
Simulated variables: conc
sim_plot(
sims4,= [:conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Predicted Concentration (ng/mL)",
ylabel = "Two Parallel first-order absorption",
title
), )
7 Weibull-Type Absorption
Yet other situations call for an absorption process that varies over time. One such model is this Weibull-type model, whereby the absorption is by a first-order process, but with a rate constant that is continuously changing over time according to a Weibull function (i.e., the CDF of a Weibull distribution). Here is the Pumas code for this model:
= @model begin
weibullabs
@param begin
∈ RealDomain(; lower = 0)
tvcl ∈ RealDomain(; lower = 0)
tvvc ∈ RealDomain(; lower = 0)
tvka ∈ RealDomain(; lower = 0)
tvγ ∈ PDiagDomain(4)
Ω end
@random begin
~ MvNormal(Ω)
η end
@covariates wt
@pre begin
= tvcl * (wt / 70)^0.75 * exp(η[1])
CL = tvvc * (wt / 70) * exp(η[2])
Vc = tvka * exp(η[3]) # Maximum Ka as t → ∞
Ka∞ = tvγ * exp(η[4]) # Controls the steepness of the Ka curve
γ = 1 - exp(-(Ka∞ * t)^γ) # Weibull function
Kaᵗ end
@dynamics begin
' = -Kaᵗ * Depot
Depot' = Kaᵗ * Depot - (CL / Vc) * Central
Centralend
@derived begin
= @. Central / Vc
conc end
end
PumasModel
Parameters: tvcl, tvvc, tvka, tvγ, Ω
Random effects: η
Covariates: wt
Dynamical system variables: Depot, Central
Dynamical system type: Nonlinear ODE
Derived: conc
Observed: conc
=
param5 = 5, tvvc = 50, tvka = 0.4, tvγ = 4, Ω = Diagonal([0.04, 0.04, 0.36, 0.04]))
(; tvcl = DosageRegimen(100; cmt = 1, time = 0)
dose5 = map(
subj_with_covariates5 -> Subject(;
i = i,
id = dose5,
events = choose_covariates(),
covariates = (; conc = nothing),
observations
),1:10,
)= simobs(weibullabs, subj_with_covariates5, param5, obstimes = 0:0.1:24) sims5
Simulated population (Vector{<:Subject})
Simulated subjects: 10
Simulated variables: conc
sim_plot(
sims5,= [:conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Predicted Concentration (ng/mL)",
ylabel = "Weibull absorption",
title
), )
8 Erlang Absorption
The Erlang absorption model is based on the concept of transit compartments. The Erlang distribution is a special case of the Gamma distribution that arises as the sum of \(k\) independent exponential distributions. This is exactly the distribution of the transit time taken by a particle passing through a sequence of \(k\) compartments where the transition from one compartment to the next is governed by first-order processes with a common rate constant \(K_{tr}\). In Pumas, this model can be written with the following code (we assume \(N = 5\) transit compartments):
= @model begin
erlangabs
@param begin
∈ RealDomain(; lower = 0)
tvcl ∈ RealDomain(; lower = 0)
tvvc ∈ RealDomain(; lower = 0)
tvktr ∈ PSDDomain(3)
Ω end
@random begin
~ MvNormal(Ω)
η end
@covariates wt
@pre begin
= tvcl * (wt / 70)^0.75 * exp(η[1])
CL = tvvc * (wt / 70) * exp(η[2])
Vc = tvktr * exp(η[3])
Ktr end
@dynamics begin
' = -Ktr * Depot
Depot' = Ktr * Depot - Ktr * Transit1
Transit1' = Ktr * Transit1 - Ktr * Transit2
Transit2' = Ktr * Transit2 - Ktr * Transit3
Transit3' = Ktr * Transit3 - Ktr * Transit4
Transit4' = Ktr * Transit4 - Ktr * Transit5
Transit5' = Ktr * Transit5 - (CL / Vc) * Central
Centralend
@observed begin
= @. Central / Vc
conc end
end
PumasModel
Parameters: tvcl, tvvc, tvktr, Ω
Random effects: η
Covariates: wt
Dynamical system variables: Depot, Transit1, Transit2, Transit3, Transit4, Transit5, Central
Dynamical system type: Matrix exponential
Derived:
Observed: conc
= (; tvcl = 7, tvvc = 32, tvktr = 2.6, Ω = Diagonal([0.09, 0.22, 0.10]))
param6 = DosageRegimen(100; cmt = 1, time = 0)
dose6 = map(
subj_with_covariates6 -> Subject(;
i = i,
id = dose6,
events = choose_covariates(),
covariates = (; conc = nothing),
observations
),1:10,
)= simobs(erlangabs, subj_with_covariates6, param6; obstimes = 0:1:24) sims6
Simulated population (Vector{<:Subject})
Simulated subjects: 10
Simulated variables: conc
sim_plot(
sims6,= [:conc],
observations = (; fontsize = 18),
figure = (;
axis = "Time (hr)",
xlabel = "Predicted Concentration (ng/mL)",
ylabel = "Erlang absorption",
title
), )
9 Conclusion
We have seen in this tutorial some examples of how to implement various absorption models that are commonly employed in pharmacokinetic modeling using Pumas. The powerful Pumas modeling language framework makes it relatively straightforward to implement these and other complex PK and PKPD models for modeling and simulation purposes.
This concludes our tutorial on basic absorption models with Pumas. Thanks for reading, and please be sure to check out our other tutorials as well.